Colormap in Matplotlib
Colormap is an important component in data visualization using Matplotlib. It represents the mapping between values and colors in a plot, helping to highlight patterns and trends in the data. In this article, we will explore various aspects of colormaps in Matplotlib and how to use them effectively in different types of plots.
Introduction to Colormaps
Colormaps are used to assign colors to different values in a plot, creating a visual representation of the data. Matplotlib provides a wide range of built-in colormaps that can be easily applied to various types of plots. Let’s start by looking at some basic colormaps and how to use them in a simple plot.
Example 1: Using the viridis
Colormap
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
y = np.sin(x)
plt.figure()
plt.scatter(x, y, c=y, cmap='viridis')
plt.colorbar()
plt.show()
Output:
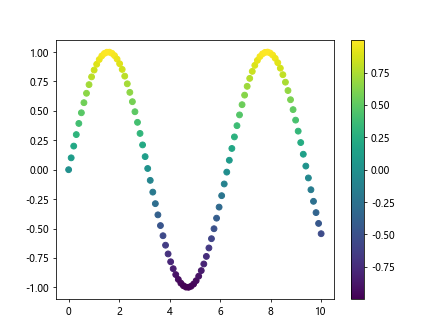
In this example, we create a scatter plot of y
values using the viridis
colormap. The c
parameter specifies the colors based on the y
values, and cmap='viridis'
sets the colormap to viridis
. The plt.colorbar()
function adds a color bar to the plot, indicating the mapping between colors and values.
Example 2: Changing Colormap with set_cmap
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
y = np.sin(x)
plt.figure()
plt.scatter(x, y, c=y, cmap='plasma')
plt.colorbar()
plt.set_cmap('plasma_r')
plt.show()
Output:
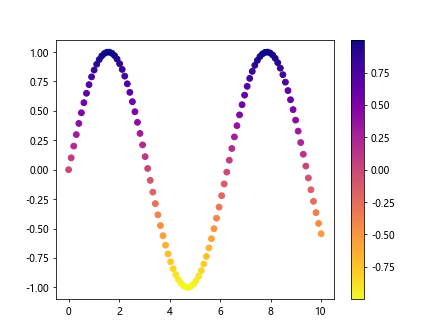
In this example, we first create a scatter plot with the plasma
colormap. Then, we change the colormap to plasma_r
using the set_cmap
function. This function allows us to dynamically change the colormap of a plot after it has been created.
List of Built-In Colormaps
Matplotlib provides a variety of built-in colormaps that can be used for different purposes. Here are some popular colormaps and their visual representations:
- Viridis
Plasma
Inferno
Magma
Cividis
Example 3: Using the inferno
Colormap
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
y = np.sin(x)
plt.figure()
plt.plot(x, y, color='blue', cmap='inferno')
plt.show()
In this example, we create a line plot with the inferno
colormap, which provides a visually appealing color scheme for the plot.