Figure Size in Matplotlib
Matplotlib is a powerful data visualization library in Python that allows you to create a wide variety of plots and charts. One important aspect of creating visually appealing plots is controlling the size of the figure. In this article, we will explore how to customize the figure size in Matplotlib.
Setting Figure Size
You can set the size of a figure in Matplotlib using the figsize
parameter in the plt.figure()
function. The figsize
parameter takes a tuple of two values representing the width and height in inches. Here’s an example:
import matplotlib.pyplot as plt
plt.figure(figsize=(10, 6))
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
plt.show()
Output:
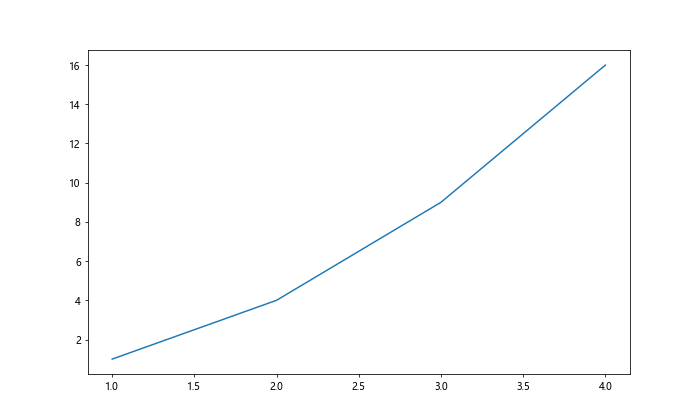
In the above example, we set the figure size to be 10 inches in width and 6 inches in height. Run the code and you will see the plot displayed with the specified figure size.
Customizing Figure Size for Subplots
You can also set the figure size for subplots in Matplotlib by passing the figsize
parameter to the plt.subplots()
function. Here’s an example with two subplots:
import matplotlib.pyplot as plt
fig, ax = plt.subplots(1, 2, figsize=(12, 6))
ax[0].plot([1, 2, 3, 4], [1, 4, 9, 16])
ax[1].plot([1, 2, 3, 4], [1, 2, 3, 4])
plt.show()
Output:
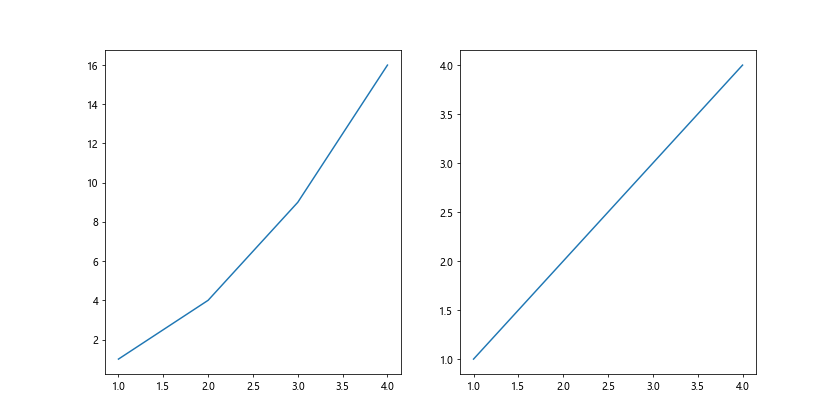
In the above example, we create a figure with two subplots, each with a size of 12 inches in width and 6 inches in height. Run the code to see the two subplots displayed side by side with the specified sizes.
Adjusting Figure Size after Creation
If you want to adjust the size of a figure after it has been created, you can use the set_size_inches()
method of the Figure
object. Here’s an example:
import matplotlib.pyplot as plt
fig = plt.figure(figsize=(8, 5))
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
# Adjust the figure size to be 10 inches in width and 6 inches in height
fig.set_size_inches(10, 6)
plt.show()
Output:
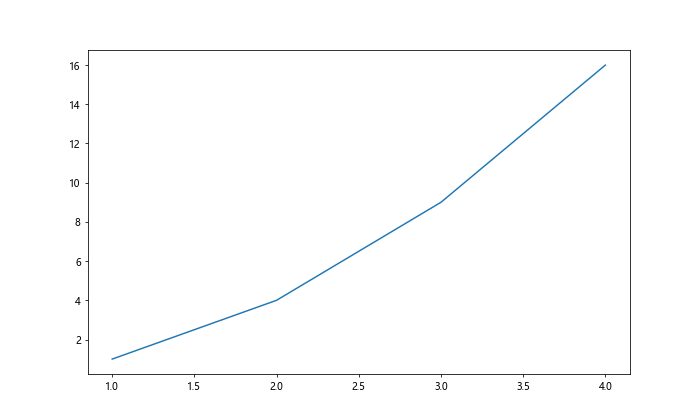
In the above example, we initially create a figure with a size of 8 inches in width and 5 inches in height. Then, we use the set_size_inches()
method to adjust the figure size to 10 inches in width and 6 inches in height. Run the code to see the plot displayed with the updated figure size.
Aspect Ratio in Figure Size
In Matplotlib, you can control the aspect ratio of a figure by setting the width and height such that the ratio between them represents the desired aspect ratio. For example, to create a figure with a specific aspect ratio, you can calculate the height based on the width and the desired aspect ratio. Here’s an example:
import matplotlib.pyplot as plt
width = 8
aspect_ratio = 16/9 # Desired aspect ratio
height = width * aspect_ratio
plt.figure(figsize=(width, height))
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
plt.show()
Output:
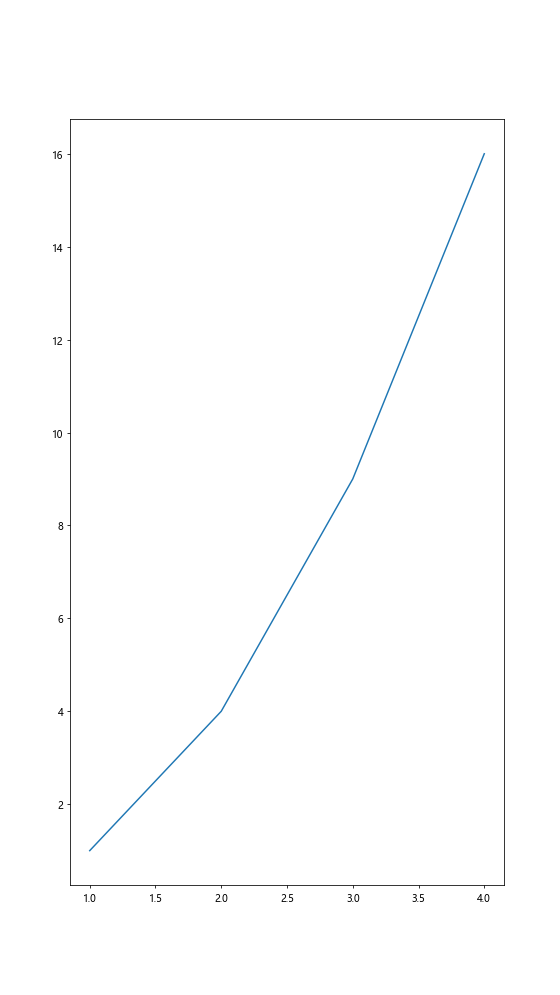
In the above example, we calculate the height based on the width and the desired aspect ratio of 16:9. The figure will be displayed with the specified aspect ratio when you run the code.
Using Figure Size in Different Types of Plots
You can customize the figure size for different types of plots in Matplotlib to ensure they are displayed optimally. Here are some examples of setting figure size for various types of plots:
Line Plot
import matplotlib.pyplot as plt
plt.figure(figsize=(8, 5))
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
plt.show()
Output:
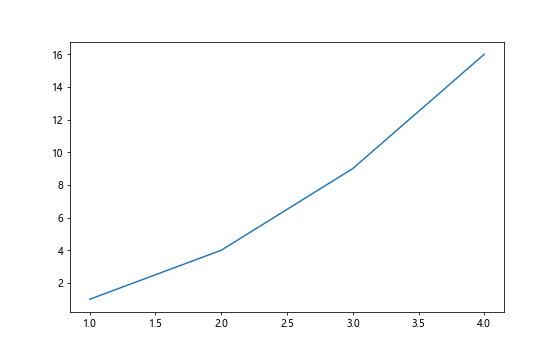
Scatter Plot
import matplotlib.pyplot as plt
plt.figure(figsize=(10, 6))
plt.scatter([1, 2, 3, 4], [1, 4, 9, 16])
plt.show()
Output:
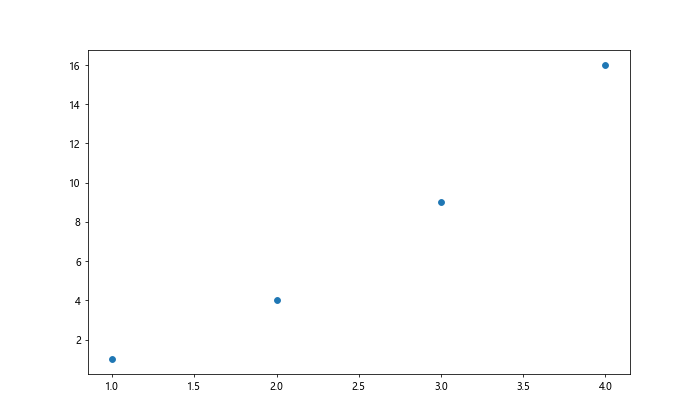
Bar Chart
import matplotlib.pyplot as plt
plt.figure(figsize=(12, 6))
plt.bar([1, 2, 3, 4], [1, 4, 9, 16])
plt.show()
Output:
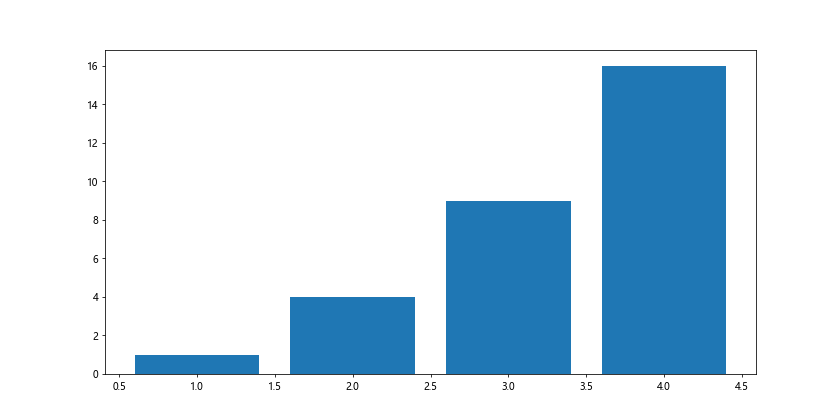
Histogram
import matplotlib.pyplot as plt
plt.figure(figsize=(8, 5))
plt.hist([1, 2, 3, 3, 4, 4, 4, 5, 5, 5, 5])
plt.show()
Output:
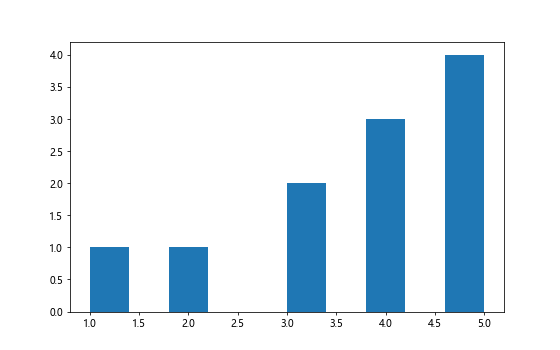
Pie Chart
import matplotlib.pyplot as plt
plt.figure(figsize=(6, 6))
plt.pie([25, 35, 20, 20], labels=['A', 'B', 'C', 'D'])
plt.show()
Output:
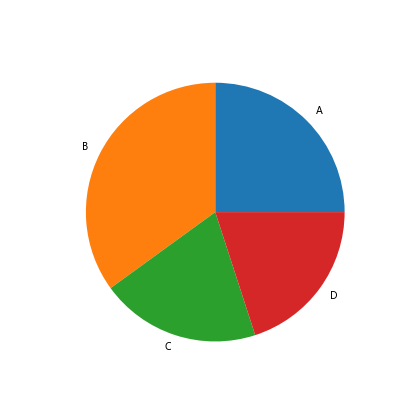
Figure Size in Matplotlib Conclusion
In this article, we have explored how to customize the figure size in Matplotlib. By setting the figure size appropriately, you can create plots and charts that are visually appealing and convey information effectively. Experiment with different figure sizes and aspect ratios to find the best display for your data visualizations.