Colorbar Vmin Vmax
When working with visualizations in matplotlib, the colorbar is a key component to help understand the data being displayed. The vmin
and vmax
parameters in the colorbar function allow us to control the range of values that are mapped to colors in the colorbar. In this article, we will explore how to use vmin
and vmax
to customize the colorbar in matplotlib.
Setting vmin and vmax values
The vmin
and vmax
parameters in the colorbar function allow us to set the minimum and maximum values that are mapped to the colors in the colorbar. By default, the colorbar will match the full range of values in the data, but we can customize this range by setting vmin
and vmax
.
import matplotlib.pyplot as plt
import numpy as np
# Create a random 2D array
data = np.random.rand(10, 10)
# Create a heatmap of the data with custom vmin and vmax
plt.imshow(data, cmap='viridis')
plt.colorbar(vmin=0.2, vmax=0.8)
plt.show()
In the example above, we create a heatmap of a random 10×10 array and set the vmin to 0.2 and vmax to 0.8. This means that only values between 0.2 and 0.8 will be mapped to colors in the colorbar.
Adjusting vmin and vmax in a scatter plot
The vmin
and vmax
parameters can also be used in scatter plots to control the color mapping. Let’s see an example of how to use vmin
and vmax
in a scatter plot.
import matplotlib.pyplot as plt
import numpy as np
# Create random x and y coordinates
x = np.random.rand(100)
y = np.random.rand(100)
# Create random colors
colors = np.random.rand(100)
# Create a scatter plot with custom vmin and vmax for colors
plt.scatter(x, y, c=colors, cmap='cool')
plt.colorbar(vmin=0.2, vmax=0.8)
plt.show()
In the scatter plot example above, we set vmin
to 0.2 and vmax
to 0.8 to map the colors to a specific range of values.
Using vmin and vmax with contour plots
Contour plots can also benefit from customizing the range of values in the colorbar. Let’s see how to use vmin
and vmax
in a contour plot.
import matplotlib.pyplot as plt
import numpy as np
# Create data for a contour plot
x = np.linspace(-2, 2, 100)
y = np.linspace(-2, 2, 100)
X, Y = np.meshgrid(x, y)
Z = np.sin(X) * np.cos(Y)
# Create a contour plot with custom vmin and vmax
plt.contourf(X, Y, Z, cmap='RdGy')
plt.colorbar(vmin=-0.5, vmax=0.5)
plt.show()
In the contour plot example above, we set vmin
to -0.5 and vmax
to 0.5 to map the colors to the specified range of values.
Setting vmin and vmax with an image plot
Image plots are another type of visualization where vmin
and vmax
can be useful. Let’s see an example of setting vmin
and vmax
in an image plot.
import matplotlib.pyplot as plt
import numpy as np
# Create a random 2D array for an image plot
data = np.random.rand(10, 10)
# Display the image with custom vmin and vmax
plt.imshow(data, cmap='inferno')
plt.colorbar(vmin=0.3, vmax=0.7)
plt.show()
In the image plot example above, we set vmin
to 0.3 and vmax
to 0.7 to map the colors to a specific range of values.
Adjusting vmin and vmax in a bar plot
Bar plots are another type of visualization where vmin
and vmax
can be used to customize the color mapping. Let’s see an example of setting vmin
and vmax
in a bar plot.
import matplotlib.pyplot as plt
import numpy as np
# Create random data for a bar plot
x = np.arange(10)
y = np.random.rand(10)
# Create a bar plot with custom vmin and vmax for colors
plt.bar(x, y, color=plt.cm.viridis((y - 0.3) / 0.4))
plt.colorbar(label="Value")
plt.show()
In the bar plot example above, we set the colors based on the values in y
and adjust the color mapping using vmin
and vmax
.
Using vmin and vmax in a pie chart
Even pie charts can benefit from customizing the color mapping with vmin
and vmax
. Let’s see an example of setting vmin
and vmax
in a pie chart.
import matplotlib.pyplot as plt
import numpy as np
# Create random data for a pie chart
sizes = np.random.randint(1, 10, 5)
# Create a pie chart with custom vmin and vmax for colors
plt.pie(sizes, colors=plt.cm.plasma((sizes - 1) / 9))
plt.colorbar(label="Value")
plt.show()
In the pie chart example above, we set the colors based on the values in sizes
and customize the color mapping using vmin
and vmax
.
Adjusting vmin and vmax in a contourf plot
The vmin
and vmax
parameters can also be used in contourf plots to control the color mapping. Let’s see an example of using vmin
and vmax
in a contourf plot.
import matplotlib.pyplot as plt
import numpy as np
# Create data for a contourf plot
x = np.linspace(-2, 2, 100)
y = np.linspace(-2, 2, 100)
X, Y = np.meshgrid(x, y)
Z = np.sin(X) * np.cos(Y)
# Create a contourf plot with custom vmin and vmax
plt.contourf(X, Y, Z, cmap='PuOr', vmin=-0.5, vmax=0.5)
plt.colorbar(label="Value")
plt.show()
Output:
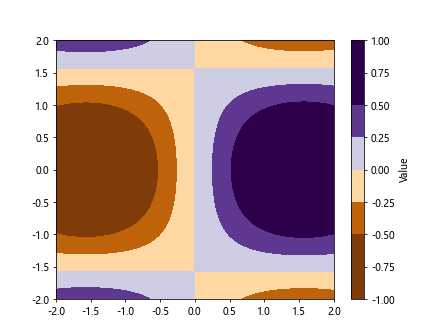
In the contourf plot example above, we set vmin
to -0.5 and vmax
to 0.5 to map the colors to the specified range of values.
Setting vmin and vmax in a quiver plot
Quiver plots can also be customized with vmin
and vmax
to control the color mapping. Let’s see an example of using vmin
and vmax
in a quiver plot.
import matplotlib.pyplot as plt
import numpy as np
# Create data for a quiver plot
X, Y = np.meshgrid(np.arange(-2, 2, 0.5), np.arange(-2, 2, 0.5))
U = -1 - X**2 + Y
V = 1 + X - Y**2
# Create a quiver plot with custom vmin and vmax
plt.quiver(X, Y, U, V, cmap='seismic', angles='xy', scale_units='xy', scale=1, vmin=-2, vmax=2)
plt.colorbar(label="Magnitude")
plt.show()
In the quiver plot example above, we set vmin
to -2 and vmax
to 2 to map the colors to the specified range of values.
Adjusting vmin and vmax in a streamplot
Streamplots can also benefit from customizing the range of values in the colorbar. Let’s see how to use vmin
and vmax
in a streamplot.
import matplotlib.pyplot as plt
import numpy as np
# Create data for a streamplot
Y, X = np.mgrid[-3:3:100j, -3:3:100j]
U = -1 - X**2 + Y
V = 1 + X - Y**2
# Create a streamplot with custom vmin and vmax
plt.streamplot(X, Y, U, V, color=U, cmap='cool', integration_direction='both', vmin=-2, vmax=2)
plt.colorbar(label="Magnitude")
plt.show()
In the streamplot example above, we set vmin
to -2 and vmax
to 2 to map the colors to the specified range of values.
Setting vmin and vmax in a hist2d plot
The vmin
and vmax
parameters can also be used in hist2d plots to control the color mapping. Let’s see an example of using vmin
and vmax
in a hist2d plot.
import matplotlib.pyplot as plt
import numpy as np
# Create random data for a hist2d plot
x = np.random.randn(1000)
y = np.random.randn(1000)
# Create a hist2d plot with custom vmin and vmax
plt.hist2d(x, y, bins=30, cmap='spring', vmin=0, vmax=100)
plt.colorbar(label="Counts")
plt.show()
Output:
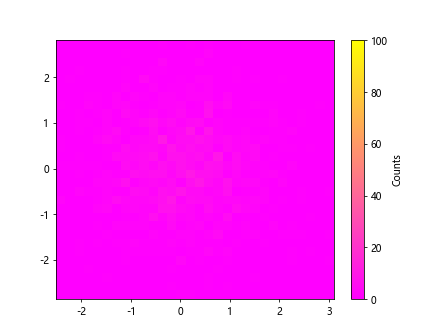
In the hist2d plot example above, we set vmin
to 0 and vmax
to 100 to map the colors to the specified range of values.
Adjusting vmin and vmax in a hexbin plot
Hexbin plots can also be customized with vmin
and vmax
to control the color mapping. Let’s see an example of using vmin
and vmax
in a hexbin plot.
import matplotlib.pyplot as plt
import numpy as np
# Create random data for a hexbin plot
x = np.random.randn(1000)
y = np.random.randn(1000)
# Create a hexbin plot with custom vmin and vmax for colors
plt.hexbin(x, y, gridsize=30, cmap='cool', vmin=0, vmax=100)
plt.colorbar(label="Counts")
plt.show()
Output:
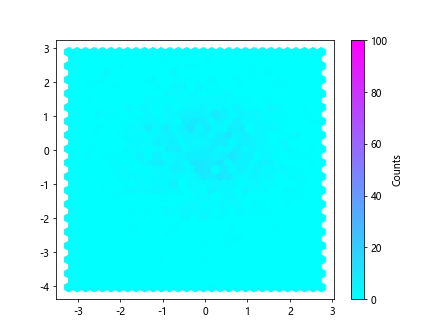
In the hexbin plot example above, we set vmin
to 0 and vmax
to 100 to map the colors to the specified range of values.
Using vmin and vmax in a barbs plot
Barbs plots can also benefit from customizing the color mapping with vmin
and vmax
. Let’s see an example of setting vmin
and vmax
in a barbs plot.
import matplotlib.pyplot as plt
import numpy as np
# Create data for a barbs plot
X, Y = np.meshgrid(np.arange(-2, 2, 0.5), np.arange(-2, 2, 0.5))
U = -1 - X**2 + Y
V = 1 + X - Y**2
# Create a barbs plot with custom vmin and vmax
plt.barbs(X, Y, U, V, color='Blue', cmap='cool', norm=None, vmin=-2, vmax=2)
plt.colorbar(label="Magnitude")
plt.show()
In the barbs plot example above, we set vmin
to -2 and vmax
to 2 to map the colors to the specified range of values.
colorbar vmin vmax Conclusion
In this article, we have explored how to use the vmin
and vmax
parameters in matplotlib’s colorbar function to customize the color mapping in various types of plots such as heatmaps, scatter plots, contour plots, image plots, bar plots, pie charts, and more. By adjusting the vmin
and vmax
values, we can control the range of values that are mapped to colors in the colorbar, allowing for more meaningful visualizations of our data. Next time you create a matplotlib plot, consider using vmin
and vmax
to enhance the clarity and impact of your visualizations.