How to Change the Label Size and Tick Label Size of Colorbar Using Matplotlib in Python
Change the label size and tick label size of colorbar using Matplotlib in Python is an essential skill for data visualization enthusiasts and professionals alike. This article will delve deep into the intricacies of manipulating colorbar labels and tick labels in Matplotlib, providing you with a thorough understanding of the subject. We’ll explore various techniques, best practices, and common pitfalls to help you master the art of customizing colorbars in your plots.
Understanding Colorbars in Matplotlib
Before we dive into changing the label size and tick label size of colorbar using Matplotlib in Python, it’s crucial to understand what colorbars are and their significance in data visualization. Colorbars are visual representations of the mapping between color and data values in a plot. They provide a legend that helps viewers interpret the meaning of colors in your visualization.
Matplotlib, a popular plotting library in Python, offers robust support for creating and customizing colorbars. Let’s start with a basic example of creating a plot with a colorbar:
import matplotlib.pyplot as plt
import numpy as np
# Create sample data
data = np.random.rand(10, 10)
# Create a figure and axis
fig, ax = plt.subplots()
# Create a heatmap with a colorbar
im = ax.imshow(data)
cbar = plt.colorbar(im)
# Set the title
plt.title("Basic Colorbar Example - how2matplotlib.com")
# Show the plot
plt.show()
Output:
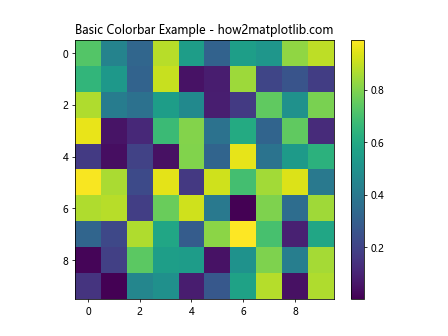
In this example, we create a simple heatmap with a colorbar. The colorbar is automatically added to the plot using plt.colorbar()
. However, you might notice that the default label size and tick label size may not always be ideal for your specific visualization needs.
Changing the Label Size of Colorbar
Change the label size and tick label size of colorbar using Matplotlib in Python is often necessary to improve the readability and aesthetics of your plots. Let’s start by focusing on changing the label size of the colorbar.
To change the label size of a colorbar, you can use the set_label()
method of the colorbar object and specify the fontsize
parameter. Here’s an example:
import matplotlib.pyplot as plt
import numpy as np
# Create sample data
data = np.random.rand(10, 10)
# Create a figure and axis
fig, ax = plt.subplots()
# Create a heatmap with a colorbar
im = ax.imshow(data)
cbar = plt.colorbar(im)
# Change the label size of the colorbar
cbar.set_label("Values - how2matplotlib.com", fontsize=16)
# Set the title
plt.title("Colorbar with Custom Label Size")
# Show the plot
plt.show()
Output:
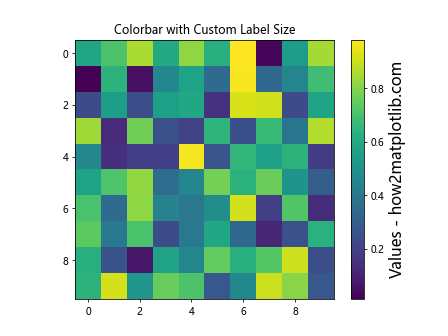
In this example, we use cbar.set_label()
to set the label text and its size. The fontsize
parameter allows you to specify the desired font size for the label.
You can also change the label size using the labelsize
parameter when creating the colorbar:
import matplotlib.pyplot as plt
import numpy as np
# Create sample data
data = np.random.rand(10, 10)
# Create a figure and axis
fig, ax = plt.subplots()
# Create a heatmap with a colorbar
im = ax.imshow(data)
cbar = plt.colorbar(im, label="Values - how2matplotlib.com", labelsize=16)
# Set the title
plt.title("Colorbar with Custom Label Size (Alternative Method)")
# Show the plot
plt.show()
This method allows you to set the label and its size in a single line of code when creating the colorbar.
Changing the Tick Label Size of Colorbar
Now that we’ve covered how to change the label size, let’s focus on changing the tick label size of colorbar using Matplotlib in Python. Tick labels are the numerical values displayed along the colorbar, indicating the range of values represented by the colors.
To change the tick label size of a colorbar, you can use the ax.tick_params()
method or modify the tick_params
property of the colorbar. Here’s an example:
import matplotlib.pyplot as plt
import numpy as np
# Create sample data
data = np.random.rand(10, 10)
# Create a figure and axis
fig, ax = plt.subplots()
# Create a heatmap with a colorbar
im = ax.imshow(data)
cbar = plt.colorbar(im)
# Change the tick label size of the colorbar
cbar.ax.tick_params(labelsize=14)
# Set the label and title
cbar.set_label("Values - how2matplotlib.com", fontsize=16)
plt.title("Colorbar with Custom Tick Label Size")
# Show the plot
plt.show()
Output:
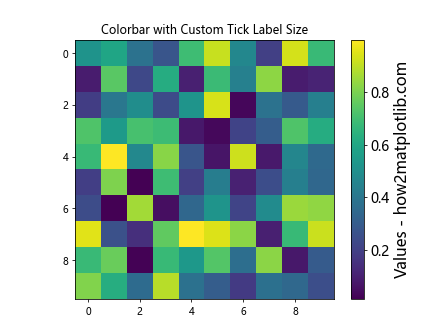
In this example, we use cbar.ax.tick_params(labelsize=14)
to change the tick label size of the colorbar. The labelsize
parameter allows you to specify the desired font size for the tick labels.
Alternatively, you can change the tick label size using the tick_params
property of the colorbar:
import matplotlib.pyplot as plt
import numpy as np
# Create sample data
data = np.random.rand(10, 10)
# Create a figure and axis
fig, ax = plt.subplots()
# Create a heatmap with a colorbar
im = ax.imshow(data)
cbar = plt.colorbar(im)
# Change the tick label size of the colorbar
cbar.ax.tick_params(axis='both', which='major', labelsize=14)
# Set the label and title
cbar.set_label("Values - how2matplotlib.com", fontsize=16)
plt.title("Colorbar with Custom Tick Label Size (Alternative Method)")
# Show the plot
plt.show()
Output:
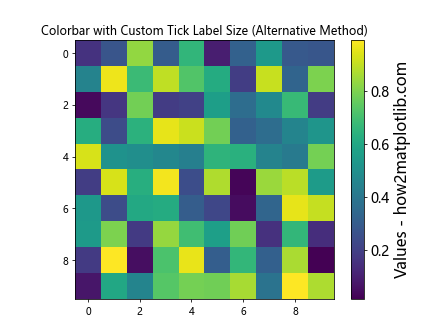
This method provides more control over the tick label size, allowing you to specify different sizes for major and minor ticks if needed.
Combining Label and Tick Label Size Changes
To change the label size and tick label size of colorbar using Matplotlib in Python simultaneously, you can combine the techniques we’ve discussed. Here’s an example that demonstrates how to change both the label size and tick label size in a single plot:
import matplotlib.pyplot as plt
import numpy as np
# Create sample data
data = np.random.rand(10, 10)
# Create a figure and axis
fig, ax = plt.subplots()
# Create a heatmap with a colorbar
im = ax.imshow(data)
cbar = plt.colorbar(im)
# Change the label size and tick label size of the colorbar
cbar.set_label("Values - how2matplotlib.com", fontsize=18)
cbar.ax.tick_params(labelsize=14)
# Set the title
plt.title("Colorbar with Custom Label and Tick Label Sizes")
# Show the plot
plt.show()
Output:
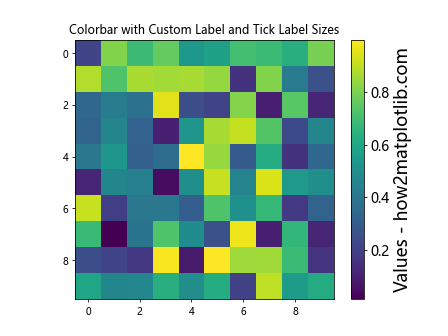
In this example, we use cbar.set_label()
to change the label size and cbar.ax.tick_params()
to change the tick label size. This approach gives you full control over both aspects of the colorbar’s appearance.
Advanced Techniques for Colorbar Customization
While changing the label size and tick label size of colorbar using Matplotlib in Python is essential, there are many other aspects of colorbar customization that you might want to explore. Let’s dive into some advanced techniques that can help you create even more polished and informative visualizations.
Changing Colorbar Orientation
By default, colorbars are displayed vertically on the right side of the plot. However, you can easily change the orientation to horizontal or place the colorbar on a different side of the plot. Here’s an example:
import matplotlib.pyplot as plt
import numpy as np
# Create sample data
data = np.random.rand(10, 10)
# Create a figure and axis
fig, ax = plt.subplots()
# Create a heatmap with a horizontal colorbar
im = ax.imshow(data)
cbar = plt.colorbar(im, orientation='horizontal', pad=0.2)
# Change the label size and tick label size of the colorbar
cbar.set_label("Values - how2matplotlib.com", fontsize=16)
cbar.ax.tick_params(labelsize=12)
# Set the title
plt.title("Horizontal Colorbar with Custom Label and Tick Label Sizes")
# Show the plot
plt.show()
Output:
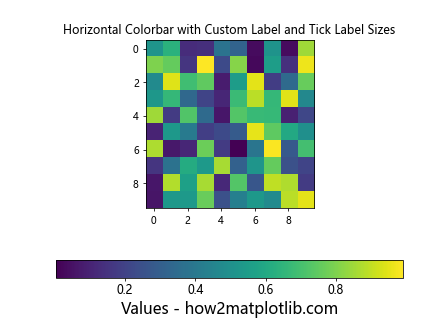
In this example, we use the orientation='horizontal'
parameter to create a horizontal colorbar. The pad
parameter adjusts the spacing between the plot and the colorbar.
Customizing Colorbar Ticks
You can also customize the number and position of ticks on your colorbar. This is particularly useful when you want to highlight specific values or ranges in your data. Here’s an example:
import matplotlib.pyplot as plt
import numpy as np
# Create sample data
data = np.random.rand(10, 10)
# Create a figure and axis
fig, ax = plt.subplots()
# Create a heatmap with a colorbar
im = ax.imshow(data, vmin=0, vmax=1)
cbar = plt.colorbar(im, ticks=[0, 0.2, 0.4, 0.6, 0.8, 1])
# Change the label size and tick label size of the colorbar
cbar.set_label("Custom Ticks - how2matplotlib.com", fontsize=16)
cbar.ax.tick_params(labelsize=12)
# Set the title
plt.title("Colorbar with Custom Ticks")
# Show the plot
plt.show()
Output:
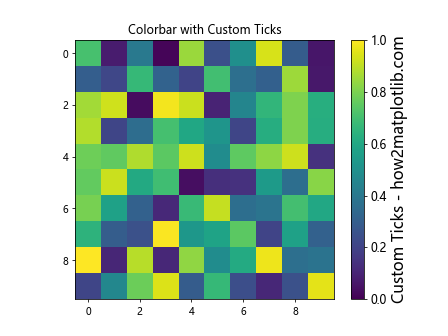
In this example, we use the ticks
parameter to specify custom tick locations on the colorbar. We also set vmin
and vmax
to ensure that the colorbar covers the full range of our data.
Using Scientific Notation for Tick Labels
When dealing with very large or very small numbers, it can be helpful to use scientific notation for the tick labels. Matplotlib provides a convenient way to do this:
import matplotlib.pyplot as plt
import numpy as np
# Create sample data
data = np.random.rand(10, 10) * 1e6
# Create a figure and axis
fig, ax = plt.subplots()
# Create a heatmap with a colorbar
im = ax.imshow(data)
cbar = plt.colorbar(im, format='%.2e')
# Change the label size and tick label size of the colorbar
cbar.set_label("Scientific Notation - how2matplotlib.com", fontsize=16)
cbar.ax.tick_params(labelsize=12)
# Set the title
plt.title("Colorbar with Scientific Notation")
# Show the plot
plt.show()
Output:
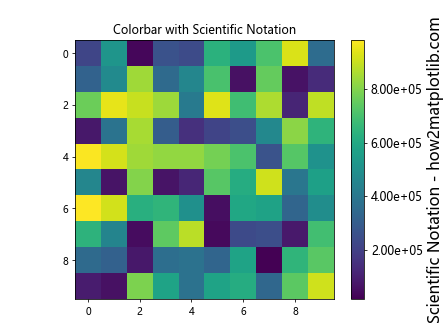
In this example, we use the format='%.2e'
parameter to display tick labels in scientific notation with two decimal places.
Best Practices for Colorbar Customization
When you change the label size and tick label size of colorbar using Matplotlib in Python, it’s important to keep some best practices in mind to ensure your visualizations are both informative and visually appealing:
- Consistency: Maintain consistent font sizes across your plot, including the main title, axis labels, and colorbar labels.
Readability: Ensure that the label and tick label sizes are large enough to be easily read, especially if your plot will be displayed on a small screen or printed in a reduced size.
Color choice: Select appropriate color maps that are both visually appealing and suitable for your data type (e.g., sequential, diverging, or qualitative).
Aspect ratio: Consider the aspect ratio of your plot when deciding on the orientation and size of your colorbar.
Tick density: Avoid overcrowding the colorbar with too many ticks, which can make it difficult to read.
Let’s implement these best practices in an example: