Adjusting Font Size of Title in Matplotlib
In this article, we will explore how to adjust the font size of the title in Matplotlib using the ax.set_title
method. The title of a plot is a crucial component as it provides context and information about the data being visualized. By adjusting the font size of the title, we can enhance the readability and aesthetics of the plot.
Examples of Changing Font Size of Title
Example 1: Setting Title Font Size to 20
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 9, 16])
ax.set_title('Title with Font Size 20', fontsize=20)
plt.show()
Output:
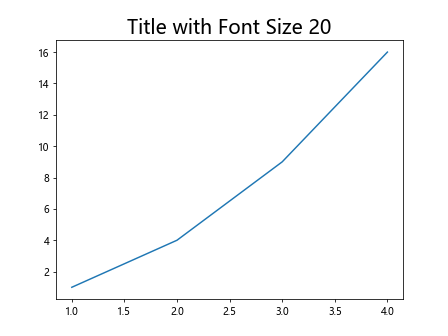
Example 2: Setting Title Font Size to 16
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 9, 16])
ax.set_title('Title with Font Size 16', fontsize=16)
plt.show()
Output:
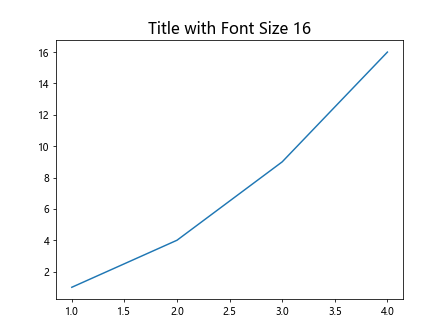
Example 3: Setting Title Font Size to 24
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 9, 16])
ax.set_title('Title with Font Size 24', fontsize=24)
plt.show()
Output:
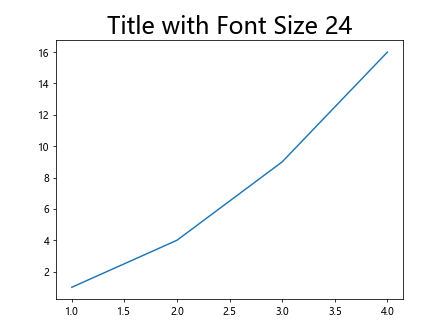
Example 4: Setting Title Font Size to 18
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 9, 16])
ax.set_title('Title with Font Size 18', fontsize=18)
plt.show()
Output:
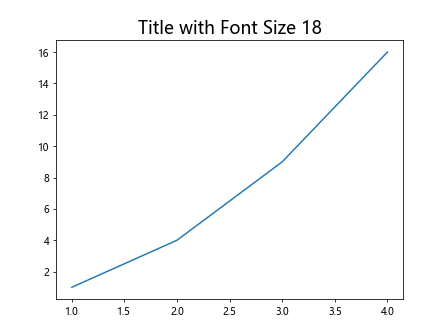
Example 5: Setting Title Font Size to 22
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 9, 16])
ax.set_title('Title with Font Size 22', fontsize=22)
plt.show()
Output:
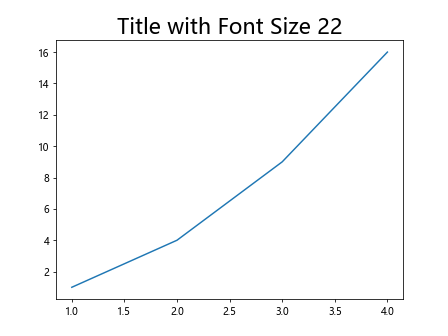
Example 6: Setting Title Font Size to 14
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 9, 16])
ax.set_title('Title with Font Size 14', fontsize=14)
plt.show()
Output:
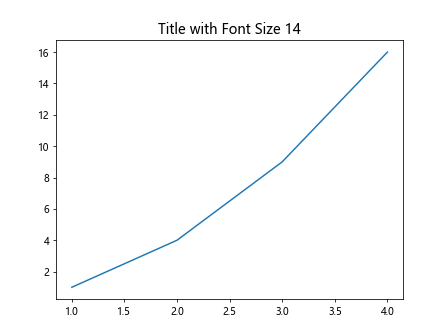
Example 7: Setting Title Font Size to 28
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 9, 16])
ax.set_title('Title with Font Size 28', fontsize=28)
plt.show()
Output:
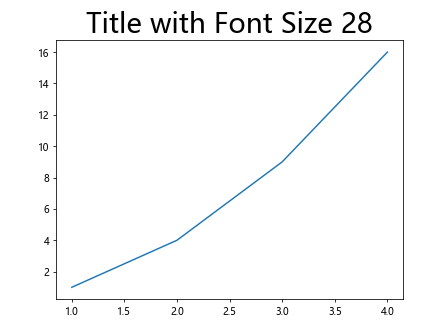
Example 8: Setting Title Font Size to 12
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 9, 16])
ax.set_title('Title with Font Size 12', fontsize=12)
plt.show()
Output:
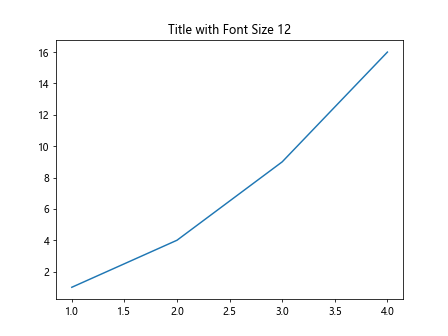
Example 9: Setting Title Font Size to 32
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 9, 16])
ax.set_title('Title with Font Size 32', fontsize=32)
plt.show()
Output:
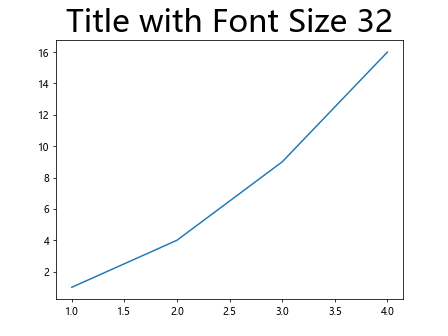
Example 10: Setting Title Font Size to 10
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 9, 16])
ax.set_title('Title with Font Size 10', fontsize=10)
plt.show()
Output:
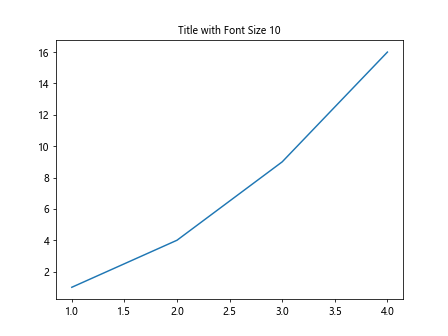
Adjusting Font Size of Title in Matplotlib Conclusion
In this article, we have explored how to adjust the font size of the title in Matplotlib using the ax.set_title
method. By changing the font size of the title, we can customize the appearance of our plots to make them more visually appealing and easier to read.