Saving Plots with ax.savefig
In data visualization, saving plots in various image formats is often necessary for presentations, reports, or publications. In Matplotlib, the savefig
method available through the ax
object allows us to save plots in different formats such as PNG, JPEG, PDF, and SVG. In this tutorial, we will explore how to use ax.savefig
to save plots in Matplotlib.
Set up a Simple Plot
Before we delve into saving plots, let’s set up a simple plot using Matplotlib. We will plot a basic line graph with random data points.
import matplotlib.pyplot as plt
import numpy as np
x = np.arange(1, 11)
y = np.random.randint(1, 10, size=10)
fig, ax = plt.subplots()
ax.plot(x, y)
plt.show()
Output:
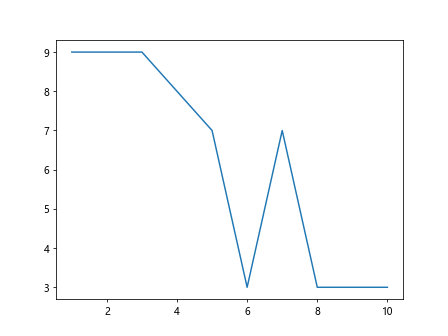
ax.savefig
” />
Save Plot as PNG
To save the plot we created as a PNG image, we can use the savefig
method on the ax
object. The following code snippet demonstrates how to save the plot as a PNG file named plot.png
.
ax.savefig('plot.png')
After running this code, you should see a file named plot.png
saved in your working directory.
Save Plot as PDF
Similarly, we can save the plot as a PDF file using the savefig
method. The code snippet below shows how to save the plot as a PDF file named plot.pdf
.
ax.savefig('plot.pdf')
Running this code will generate a file named plot.pdf
with the plot saved in PDF format.
Save Plot as JPEG
If you need to save the plot as a JPEG image, you can specify the file extension as .jpg
in the savefig
method. The following code saves the plot as a JPEG file named plot.jpg
.
ax.savefig('plot.jpg')
Executing this code will save the plot in JPEG format as plot.jpg
.
Save Plot as SVG
To save the plot as a Scalable Vector Graphics (SVG) file, you can specify the file extension as .svg
in the savefig
method. The code snippet below demonstrates saving the plot as an SVG file named plot.svg
.
ax.savefig('plot.svg')
Upon running this code, a file named plot.svg
will be created with the plot saved in SVG format.
Save Plot with Custom Size
You can also customize the size of the saved plot by specifying the dpi
(dots per inch) and figsize
parameters when calling the savefig
method. The code below shows how to save the plot with a custom size of 300 DPI and a figure size of 8×6 inches.
ax.savefig('plot_custom_size.png', dpi=300, figsize=(8, 6))
By running this code, a plot with the specified custom size will be saved as plot_custom_size.png
.
Save Plot with Transparent Background
If you want to save the plot with a transparent background, you can set the transparent
parameter to True
in the savefig
method. The code snippet below demonstrates saving the plot with a transparent background as plot_transparent.png
.
ax.savefig('plot_transparent.png', transparent=True)
Executing this code will save the plot with a transparent background as plot_transparent.png
.
Save Plot with Custom Resolution
You can adjust the resolution of the saved plot by specifying the quality
parameter for JPEG files and the compresslevel
parameter for PNG files. The code below saves the plot with a custom resolution of 90 for JPEG files and 9 for PNG files.
ax.savefig('plot_custom_res.jpg', quality=90) # for JPEG
ax.savefig('plot_custom_res.png', compresslevel=9) # for PNG
Running this code will save the plot with the specified custom resolution for JPEG and PNG files.
Save Multiple Plots in a Loop
If you need to save multiple plots in a loop, you can create a list of plots and then loop through them to save each plot individually. The following example demonstrates how to save multiple plots in a loop.
for i in range(5):
fig, ax = plt.subplots()
x = np.arange(1, 11)
y = np.random.randint(1, 10, size=10)
ax.plot(x, y)
ax.savefig(f'plot_{i}.png')
By running this code, five plots named plot_0.png
, plot_1.png
, plot_2.png
, plot_3.png
, and plot_4.png
will be saved in the working directory.
Save Plot in a Specific Directory
To save the plot in a specific directory, you can provide the full path including the directory where you want to save the plot. The code snippet below demonstrates saving the plot in a specific directory named plots
.
ax.savefig('plots/plot_directory.png')
Executing this code will save the plot in the plots
directory as plot_directory.png
.
Save Plot in Landscape Orientation
If you need to save the plot in landscape orientation, you can set the orientation
parameter to landscape
in the savefig
method. The code below shows how to save the plot in landscape orientation as plot_landscape.png
.
ax.savefig('plot_landscape.png', orientation='landscape')
Upon running this code, the plot will be saved in landscape orientation as plot_landscape.png
.
Save Plot in Portrait Orientation
Similarly, you can save the plot in portrait orientation by setting the orientation
parameter to portrait
in the savefig
method. The code snippet below demonstrates saving the plot in portrait orientation as plot_portrait.png
.
ax.savefig('plot_portrait.png', orientation='portrait')
After running this code, the plot will be saved in portrait orientation as plot_portrait.png
.
Save Plot with Custom Background Color
You can specify a custom background color for the saved plot using the facecolor
parameter in the savefig
method. The code snippet below shows how to save the plot with a custom background color of light gray.
ax.savefig('plot_custom_bg.png', facecolor='lightgrey')
Upon executing this code, the plot will be saved with a light gray background as plot_custom_bg.png
.
Save Plot with Tight Layout
To save the plot with tight layout to remove extra white spaces around the plot, you can call the tight_layout
method before saving the plot using savefig
. The code below demonstrates saving the plot with tight layout as plot_tight_layout.png
.
fig.tight_layout()
ax.savefig('plot_tight_layout.png')
By running this code, the plot will be saved with tight layout as plot_tight_layout.png
.
Save Plot with Different Line Styles
You can save the plot with different line styles by specifying the linestyle
parameter when plotting the lines. The code snippet below shows how to save the plot with dashed lines and dotted lines.
# Dashed line
ax.plot(x, y, linestyle='--')
ax.savefig('plot_dashed.png')
# Dotted line
ax.plot(x, y, linestyle=':')
ax.savefig('plot_dotted.png')
Running this code will save the plot with dashed lines as plot_dashed.png
and with dotted lines as plot_dotted.png
.
Save Plot with Marker Styles
Similarly, you can save the plot with different marker styles by setting the marker
parameter when plotting the lines. The following example demonstrates saving the plot with circle markers and square markers.
# Circle markers
ax.plot(x, y, marker='o')
ax.savefig('plot_circle_markers.png')
# Square markers
ax.plot(x, y, marker='s')
ax.savefig('plot_square_markers.png')
Executing this code will save the plot with circle markers as plot_circle_markers.png
and with square markers as plot_square_markers.png
.
Save Plot with Custom Title and Labels
To save the plot with custom titles and labels, you can set the titles for the plot and axes using the set_title
, set_xlabel
, and set_ylabel
methods. The code below demonstrates saving the plot with custom titles and labels.
# Set custom title
ax.set_title('Custom Title', fontsize=16)
# Set custom x-axis label
ax.set_xlabel('Custom X Label', fontsize=12)
# Set custom y-axis label
ax.set_ylabel('Custom Y Label', fontsize=12)
# Save the plot with custom title and labels
ax.savefig('plot_custom_title_labels.png')
Running this code will save the plot with a custom title and labels as plot_custom_title_labels.png
.
Save Plot with Grid Lines
If you want to save the plot with grid lines, you can enable them by setting the grid
parameter to True
in the savefig
method. The code snippet below demonstrates saving the plot with grid lines enabled.
ax.savefig('plot_with_grid.png', grid=True)
Executing this code will save the plot with grid lines as plot_with_grid.png
.
Save Plot with Legend
To save the plot with a legend, you can add a legend to the plot using the legend
method before saving the plot with savefig
. The code below shows how to save the plot with a legend as plot_with_legend.png
.
ax.plot(x, y, label='Data')
ax.legend()
ax.savefig('plot_with_legend.png')
By running this code, the plot will be saved with a legend as plot_with_legend.png
.
Save Specific Part of the Plot
If you only want to save a specific part of the plot, you can adjust the plot limits using the set_xlim
and set_ylim
methods before saving the plot. The code below demonstrates saving a specific part of the plot with custom limits.
ax.set_xlim(3, 8) # Set x-axis limits
ax.set_ylim(2, 7) # Set y-axis limits
ax.savefig('plot_specific_part.png')
Upon executing this code, the specific part of the plot within the specified limits will be saved as plot_specific_part.png
.
Save Plot with Annotations
You can save the plot with annotations by adding text or arrows to specific data points using the annotate
method. The code snippet below demonstrates saving the plot with annotations.
# Annotate a data point
ax.annotate('Max', xy=(x[y.argmax()], y.max()), xytext=(7, 8),
arrowprops=dict(facecolor='black', shrink=0.05))
ax.savefig('plot_with_annotation.png')
Running this code will save the plot with the specified annotation as plot_with_annotation.png
.
Save 3D Plot
If you are working with 3D plots, you can save them using the same savefig
method. The code snippet below shows how to save a 3D plot using Matplotlib.
from mpl_toolkits.mplot3d import Axes3D
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
x = np.random.normal(size=100)
y = np.random.normal(size=100)
z = np.random.normal(size=100)
ax.scatter(x, y, z)
ax.savefig('3d_plot.png')
Executing this code will save the 3D plot as 3d_plot.png
.
Conclusion
In this tutorial, we have explored how to use ax.savefig
in Matplotlib to save plots in various image formats. We have covered different aspects of saving plots, including saving plots in PNG, PDF, JPEG, and SVG formats, customizing plot size, resolution, orientation, background color, line styles, marker styles, titles, labels, grid lines, legends, annotations, and specific parts of the plot. By mastering the ax.savefig
method, you can efficiently save and export your plots for different purposes.