How to Assign the Same Label to Two Different Markers in Matplotlib
Assigning the same label to two different markers in Matplotlib is a useful technique for creating clear and informative visualizations. This article will explore various methods and best practices for assigning the same label to two different markers using Matplotlib, a powerful plotting library in Python. We’ll cover different scenarios, provide easy-to-understand examples, and offer tips to enhance your data visualization skills.
Understanding the Importance of Assigning the Same Label to Two Different Markers
Assigning the same label to two different markers is crucial when you want to represent multiple data points or series with the same meaning or category. This technique is particularly useful in scenarios such as:
- Comparing different datasets with the same classification
- Highlighting specific data points across multiple series
- Creating legends that group related data points
- Simplifying complex visualizations with multiple markers
By assigning the same label to two different markers, you can create more intuitive and easier-to-read plots, making it simpler for your audience to interpret the data.
Basic Concepts of Assigning the Same Label to Two Different Markers
Before diving into specific examples, let’s review some fundamental concepts related to assigning the same label to two different markers in Matplotlib:
- Markers: Symbols used to represent individual data points on a plot
- Labels: Text descriptions associated with markers or lines in a plot
- Legend: A key that explains the meaning of different markers, colors, or lines in a plot
When assigning the same label to two different markers, we’re essentially telling Matplotlib to group these markers under a single category in the legend, even if they appear differently on the plot.
Methods for Assigning the Same Label to Two Different Markers
There are several approaches to assigning the same label to two different markers in Matplotlib. Let’s explore some of the most common methods:
1. Using Multiple Plot Calls with the Same Label
One straightforward method is to use multiple plot()
calls with the same label. Here’s an example:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y1 = [2, 4, 6, 8, 10]
y2 = [1, 3, 5, 7, 9]
plt.figure(figsize=(10, 6))
plt.plot(x, y1, 'ro', label='Data from how2matplotlib.com')
plt.plot(x, y2, 'bs', label='Data from how2matplotlib.com')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Assigning the Same Label to Two Different Markers')
plt.legend()
plt.show()
Output:
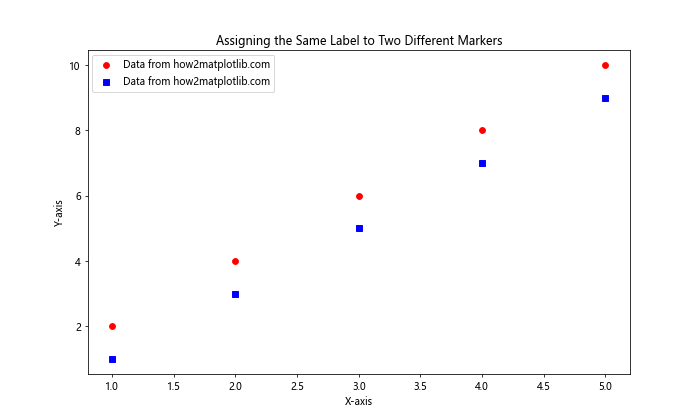
In this example, we create two separate plot()
calls with different marker styles (‘ro’ for red circles and ‘bs’ for blue squares) but assign the same label to both. This results in a single legend entry representing both markers.
2. Using a Single Plot Call with Multiple Formatting Options
Another approach is to use a single plot()
call with multiple formatting options. Here’s an example:
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 50)
y1 = np.sin(x)
y2 = np.cos(x)
plt.figure(figsize=(10, 6))
plt.plot(x, y1, 'ro', x, y2, 'bs', label='Data from how2matplotlib.com')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Assigning the Same Label to Two Different Markers')
plt.legend()
plt.show()
Output:
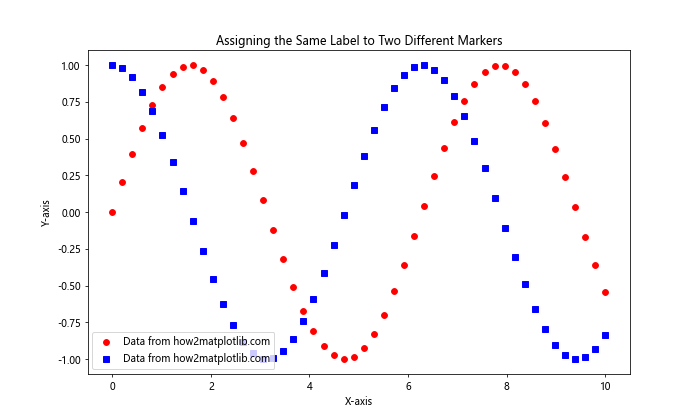
In this example, we use a single plot()
call to create two different marker styles with the same label. This method is more concise and achieves the same result as the previous example.
3. Using Scatter Plots with the Same Label
When working with scatter plots, you can use the scatter()
function to assign the same label to different markers. Here’s an example:
import matplotlib.pyplot as plt
import numpy as np
x = np.random.rand(20)
y1 = np.random.rand(20)
y2 = np.random.rand(20)
plt.figure(figsize=(10, 6))
plt.scatter(x, y1, c='red', marker='o', label='Data from how2matplotlib.com')
plt.scatter(x, y2, c='blue', marker='s', label='Data from how2matplotlib.com')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Assigning the Same Label to Two Different Markers')
plt.legend()
plt.show()
Output:
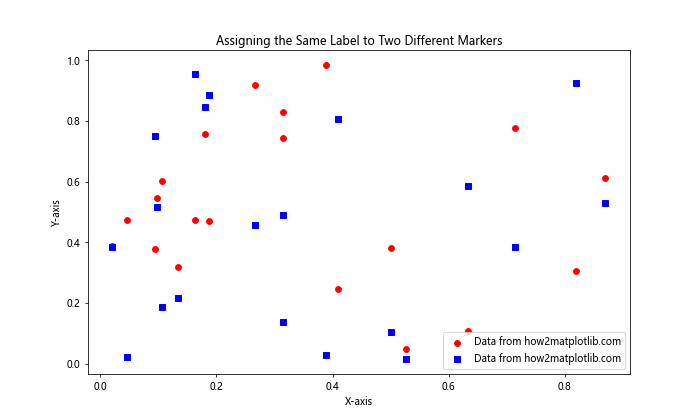
This example demonstrates how to use scatter()
to create two different marker styles with the same label in a scatter plot.
Advanced Techniques for Assigning the Same Label to Two Different Markers
Now that we’ve covered the basics, let’s explore some more advanced techniques for assigning the same label to two different markers in Matplotlib.
1. Using Custom Legend Entries
Sometimes, you may want to create a custom legend that combines different markers under the same label. Here’s an example of how to achieve this:
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 50)
y1 = np.sin(x)
y2 = np.cos(x)
fig, ax = plt.subplots(figsize=(10, 6))
line1 = ax.plot(x, y1, 'ro', label='_nolegend_')
line2 = ax.plot(x, y2, 'bs', label='_nolegend_')
# Create a custom legend entry
custom_lines = [plt.Line2D([0], [0], color='red', marker='o', linestyle=''),
plt.Line2D([0], [0], color='blue', marker='s', linestyle='')]
ax.legend(custom_lines, ['Data from how2matplotlib.com'])
ax.set_xlabel('X-axis')
ax.set_ylabel('Y-axis')
ax.set_title('Assigning the Same Label to Two Different Markers')
plt.show()
Output:
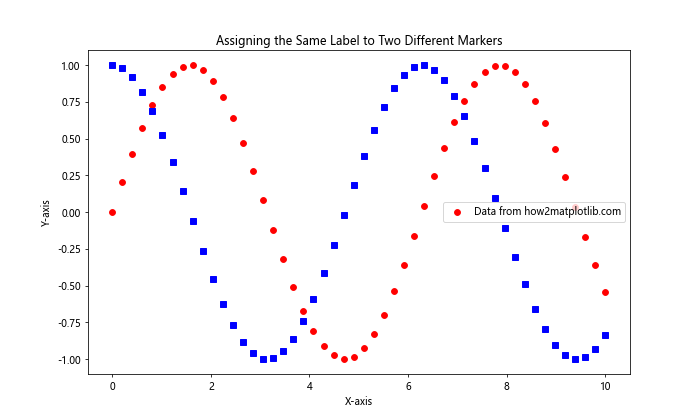
In this example, we create custom legend entries using plt.Line2D()
objects and assign them the same label. This gives us more control over how the legend appears.
2. Using a Proxy Artist for Legend Entries
Another advanced technique is to use a proxy artist to create legend entries. This is particularly useful when you want to combine different plot types under the same label:
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 50)
y1 = np.sin(x)
y2 = np.cos(x)
fig, ax = plt.subplots(figsize=(10, 6))
ax.plot(x, y1, 'ro')
ax.plot(x, y2, 'bs')
# Create proxy artists for the legend
red_circle = plt.Line2D([0], [0], marker='o', color='red', linestyle='')
blue_square = plt.Line2D([0], [0], marker='s', color='blue', linestyle='')
# Add the proxy artists to the legend
ax.legend([red_circle, blue_square], ['Data from how2matplotlib.com'])
ax.set_xlabel('X-axis')
ax.set_ylabel('Y-axis')
ax.set_title('Assigning the Same Label to Two Different Markers')
plt.show()
Output:
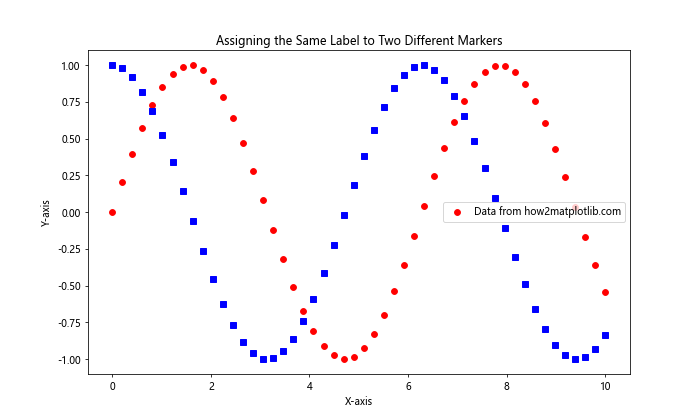
This example demonstrates how to use proxy artists to create a legend entry that represents both markers under the same label.
3. Combining Different Plot Types with the Same Label
Sometimes, you may want to assign the same label to different plot types, such as a line plot and a scatter plot. Here’s an example of how to achieve this:
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 50)
y1 = np.sin(x)
y2 = np.cos(x)
fig, ax = plt.subplots(figsize=(10, 6))
ax.plot(x, y1, 'r-', label='Data from how2matplotlib.com')
ax.scatter(x[::5], y2[::5], c='b', marker='s', label='Data from how2matplotlib.com')
ax.set_xlabel('X-axis')
ax.set_ylabel('Y-axis')
ax.set_title('Assigning the Same Label to Different Plot Types')
ax.legend()
plt.show()
Output:
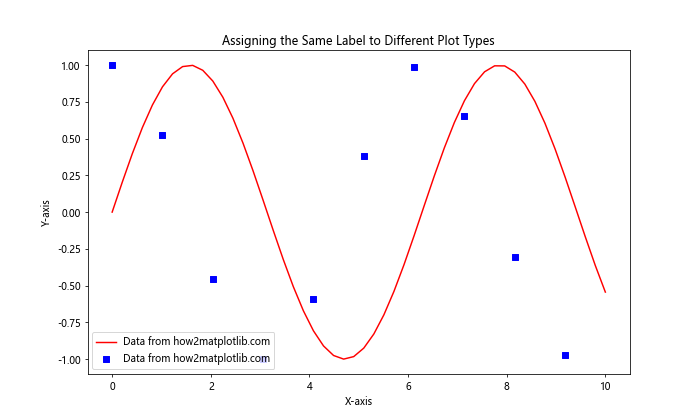
In this example, we combine a line plot and a scatter plot, assigning them the same label. This technique is useful when you want to represent different aspects of the same data series.
Best Practices for Assigning the Same Label to Two Different Markers
When assigning the same label to two different markers in Matplotlib, it’s important to follow some best practices to ensure your visualizations are clear and effective:
- Use consistent colors: When assigning the same label to different markers, try to use consistent colors to reinforce the relationship between the data points.
Choose distinct marker styles: Select marker styles that are easily distinguishable from each other to avoid confusion.
Keep labels concise: Use short, descriptive labels that clearly convey the meaning of the data points.
Limit the number of markers: Avoid using too many different markers with the same label, as this can make the plot cluttered and hard to read.
Consider using a legend title: If you’re grouping multiple markers under the same label, consider adding a legend title to provide context.
Let’s see an example that incorporates these best practices: