How can I move a tick label without moving corresponding tick in Matplotlib
In Matplotlib, the visualization library for Python, tick labels are the labels used to describe the ticks on the axes of a plot. Sometimes, it may be necessary to move these labels without altering the position of the ticks themselves. This can be useful for improving the readability of the plots, avoiding overlaps of labels, or simply for aesthetic adjustments. This article will guide you through various methods to achieve this, using detailed examples.
Introduction to Matplotlib
Matplotlib is a comprehensive library for creating static, animated, and interactive visualizations in Python. It provides an object-oriented API for embedding plots into applications using general-purpose GUI toolkits like Tkinter, wxPython, Qt, or GTK. Before diving into the specifics of moving tick labels, it’s important to understand the basic components of a Matplotlib plot.
Basic Plot Setup
Before manipulating tick labels, you first need to set up a basic plot. Here’s how you can do it:
import matplotlib.pyplot as plt
# Data
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
# Plot
plt.figure(figsize=(10, 5))
plt.plot(x, y, marker='o')
plt.title('Basic Plot - how2matplotlib.com')
plt.xlabel('X Axis')
plt.ylabel('Y Axis')
plt.show()
Output:
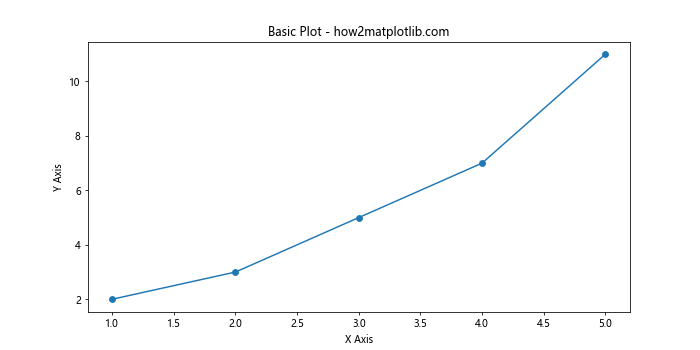
Moving Tick Labels Vertically
To move the tick labels vertically without affecting the ticks, you can adjust the set_yticklabels
method with the verticalalignment
or va
parameter.
import matplotlib.pyplot as plt
# Data
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
# Plot
plt.figure(figsize=(10, 5))
plt.plot(x, y, marker='o')
plt.title('Vertical Label Adjustment - how2matplotlib.com')
plt.xlabel('X Axis')
plt.ylabel('Y Axis')
# Adjusting tick labels
plt.gca().set_yticklabels(plt.gca().get_yticks(), verticalalignment='bottom')
plt.show()
Moving Tick Labels Horizontally
Similarly, to move the tick labels horizontally, use the horizontalalignment
or ha
parameter.
import matplotlib.pyplot as plt
# Data
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
# Plot
plt.figure(figsize=(10, 5))
plt.plot(x, y, marker='o')
plt.title('Horizontal Label Adjustment - how2matplotlib.com')
plt.xlabel('X Axis')
plt.ylabel('Y Axis')
# Adjusting tick labels
plt.gca().set_xticklabels(plt.gca().get_xticks(), horizontalalignment='right')
plt.show()
Using set_tick_params
Method
The set_tick_params
method allows for more fine-grained control over the appearance of tick labels, including their alignment.
import matplotlib.pyplot as plt
# Data
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
# Plot
plt.figure(figsize=(10, 5))
plt.plot(x, y, marker='o')
plt.title('Tick Params Adjustment - how2matplotlib.com')
plt.xlabel('X Axis')
plt.ylabel('Y Axis')
# Adjusting tick labels
plt.gca().tick_params(axis='x', labelrotation=45)
plt.gca().tick_params(axis='y', labelcolor='red')
plt.show()
Output:
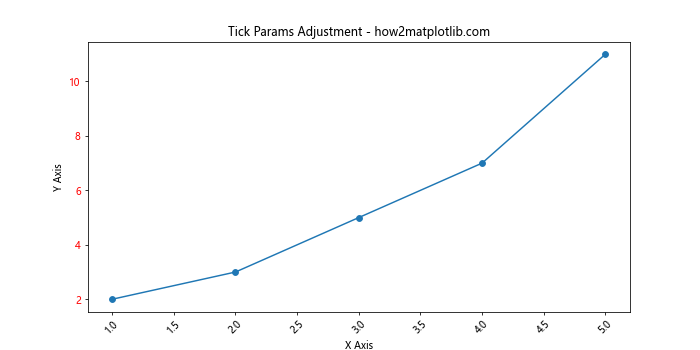
Adjusting Tick Label Padding
Padding can be adjusted to move the tick labels closer or further from the axis.
import matplotlib.pyplot as plt
# Data
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
# Plot
plt.figure(figsize=(10, 5))
plt.plot(x, y, marker='o')
plt.title('Tick Label Padding Adjustment - how2matplotlib.com')
plt.xlabel('X Axis')
plt.ylabel('Y Axis')
# Adjusting tick labels
plt.gca().tick_params(axis='x', pad=15)
plt.gca().tick_params(axis='y', pad=20)
plt.show()
Output:
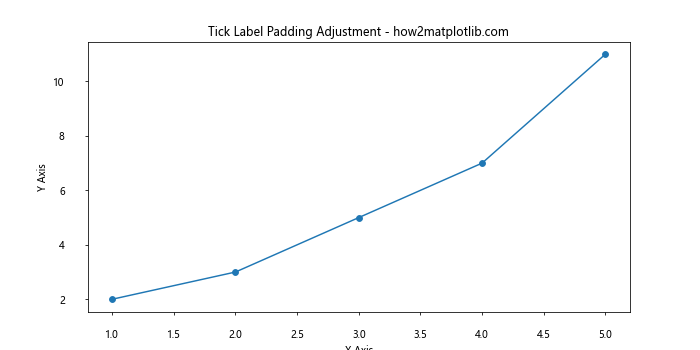
Using Text Properties
You can also use text properties to move the tick labels by specifying offsets.
import matplotlib.pyplot as plt
# Data
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
# Plot
plt.figure(figsize=(10, 5))
plt.plot(x, y, marker='o')
plt.title('Using Text Properties - how2matplotlib.com')
plt.xlabel('X Axis')
plt.ylabel('Y Axis')
# Adjusting tick labels
for label in plt.gca().get_xticklabels():
label.set_fontsize(14)
label.set_color('green')
label.set_rotation(45)
label.set_horizontalalignment('right')
plt.show()
Output:
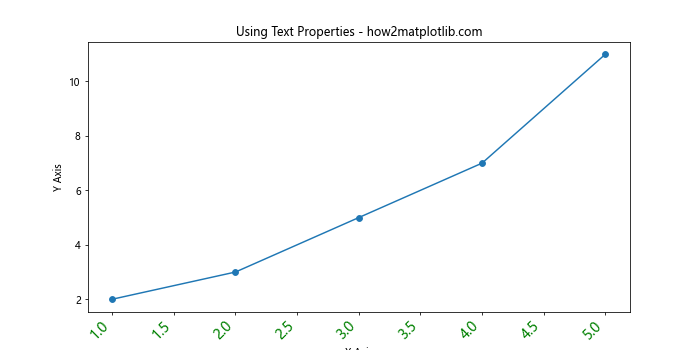
Conclusion
In this article, we explored several methods to move tick labels in Matplotlib without affecting the position of the ticks. These techniques include adjusting alignment, padding, and using text properties. Each method serves different needs and can be used to enhance the readability and aesthetics of a plot. By understanding these tools, you can create more polished and professional visualizations.