How can I make a simple 3D line with Matplotlib
Creating 3D lines with Matplotlib is a straightforward process that can add depth and visual appeal to your data visualizations. Matplotlib, a comprehensive library for creating static, animated, and interactive visualizations in Python, offers extensive support for 3D plotting, including simple 3D lines. In this article, we’ll explore how to create simple 3D lines using Matplotlib, providing detailed examples to guide you through various customization options.
Getting Started with 3D Plotting in Matplotlib
Before diving into the examples, ensure you have Matplotlib installed in your environment. If not, you can install it using pip:
pip install matplotlib
To create 3D plots, we’ll also need to import the Axes3D
class from mpl_toolkits.mplot3d
. This toolkit is a part of Matplotlib and doesn’t require separate installation.
Example 1: Basic 3D Line
Let’s start with a basic example of a 3D line. This example will plot a simple 3D line from a set of x, y, and z coordinates.
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
x = [1, 2, 3, 4, 5]
y = [5, 6, 2, 3, 13]
z = [2, 3, 3, 3, 5]
ax.plot(x, y, z, label='3D line how2matplotlib.com')
ax.legend()
plt.show()
Output:
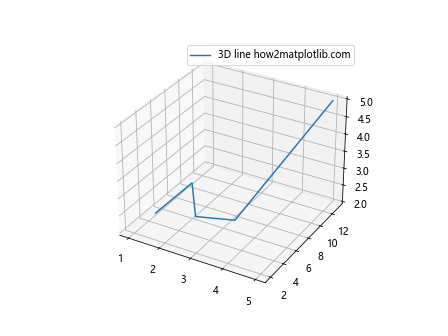
Example 2: Adding Color and Line Style
You can customize the appearance of your 3D line by changing its color and style.
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
x = [1, 2, 3, 4, 5]
y = [5, 6, 2, 3, 13]
z = [2, 3, 3, 3, 5]
ax.plot(x, y, z, color='red', linestyle='dashed', label='3D line how2matplotlib.com')
ax.legend()
plt.show()
Output:
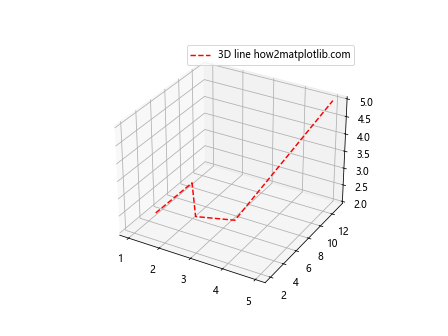
Example 3: 3D Line with Markers
Adding markers to your 3D line can help highlight specific data points.
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
x = [1, 2, 3, 4, 5]
y = [5, 6, 2, 3, 13]
z = [2, 3, 3, 3, 5]
ax.plot(x, y, z, marker='o', label='3D line how2matplotlib.com')
ax.legend()
plt.show()
Output:
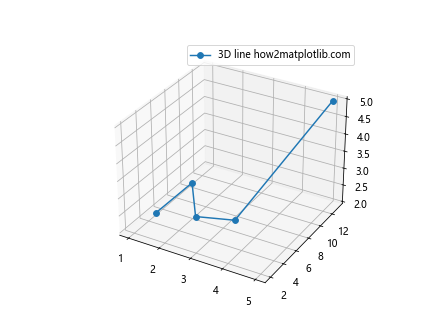
Example 4: Customizing Marker Size and Color
You can further customize the markers by adjusting their size and color.
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
x = [1, 2, 3, 4, 5]
y = [5, 6, 2, 3, 13]
z = [2, 3, 3, 3, 5]
ax.plot(x, y, z, marker='o', markersize=8, markerfacecolor='yellow', label='3D line how2matplotlib.com')
ax.legend()
plt.show()
Output:
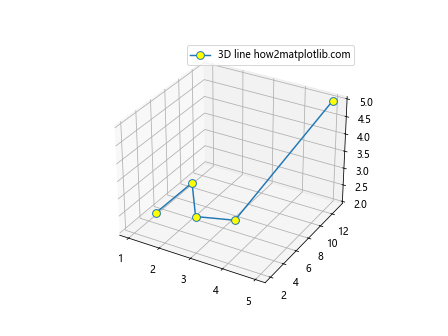
Example 5: 3D Line with Different Widths
Adjusting the line width can help make your 3D line more prominent.
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
x = [1, 2, 3, 4, 5]
y = [5, 6, 2, 3, 13]
z = [2, 3, 3, 3, 5]
ax.plot(x, y, z, linewidth=2, label='3D line how2matplotlib.com')
ax.legend()
plt.show()
Output:
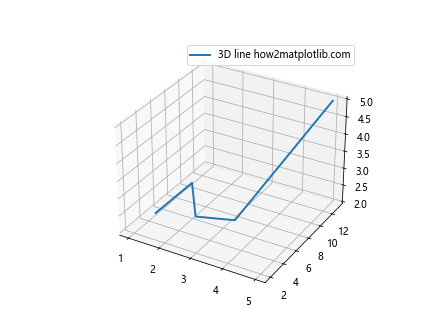
Example 6: Combining Multiple 3D Lines in One Plot
You can plot multiple 3D lines in the same figure to compare different data sets.
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
# First line
x1 = [1, 2, 3, 4, 5]
y1 = [5, 6, 2, 3, 13]
z1 = [2, 3, 3, 3, 5]
# Second line
x2 = [2, 3, 4, 5, 6]
y2 = [3, 4, 5, 6, 7]
z2 = [1, 2, 3, 4, 5]
ax.plot(x1, y1, z1, label='First 3D line how2matplotlib.com')
ax.plot(x2, y2, z2, label='Second 3D line how2matplotlib.com')
ax.legend()
plt.show()
Output:
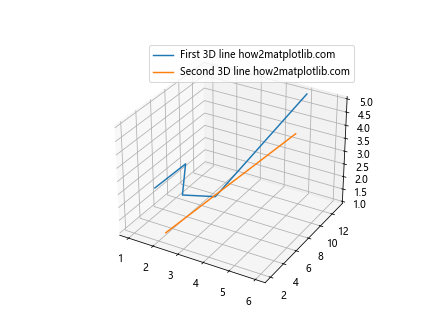
Example 7: 3D Line with Custom Tick Labels
Customizing the tick labels of your axes can help make your plot more informative.
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
x = [1, 2, 3, 4, 5]
y = [5, 6, 2, 3, 13]
z = [2, 3, 3, 3, 5]
ax.plot(x, y, z, label='3D line how2matplotlib.com')
ax.set_xticks([1, 3, 5])
ax.set_yticks([3, 6, 9, 12])
ax.set_zticks([1, 2, 3, 4, 5])
ax.legend()
plt.show()
Output:
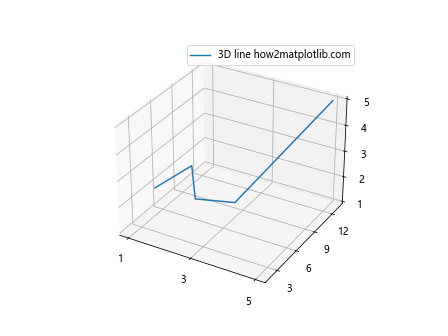
Example 8: Adding a Grid to the 3D Plot
A grid can improve the readability of your 3D plot by providing a reference for the data points.
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
x = [1, 2, 3, 4, 5]
y = [5, 6, 2, 3, 13]
z = [2, 3, 3, 3, 5]
ax.plot(x, y, z, label='3D line how2matplotlib.com')
ax.grid(True)
ax.legend()
plt.show()
Output:
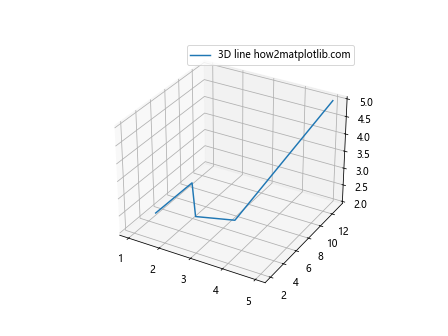
Example 9: Setting the Title and Labels
Adding a title and axis labels can provide context to your 3D plot.
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
x = [1, 2, 3, 4, 5]
y = [5, 6, 2, 3, 13]
z = [2, 3, 3, 3, 5]
ax.plot(x, y, z, label='3D line how2matplotlib.com')
ax.set_title('3D Line Plot Example')
ax.set_xlabel('X axis')
ax.set_ylabel('Y axis')
ax.set_zlabel('Z axis')
ax.legend()
plt.show()
Output:
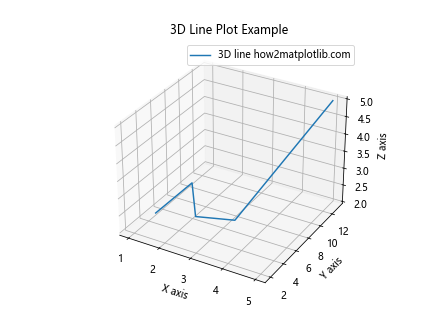
Example 10: Rotating the View Angle
Adjusting the view angle can help you visualize the 3D line from different perspectives.
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
x = [1, 2, 3, 4, 5]
y = [5, 6, 2, 3, 13]
z = [2, 3, 3, 3, 5]
ax.plot(x, y, z, label='3D line how2matplotlib.com')
ax.view_init(elev=20., azim=30)
ax.legend()
plt.show()
Output:
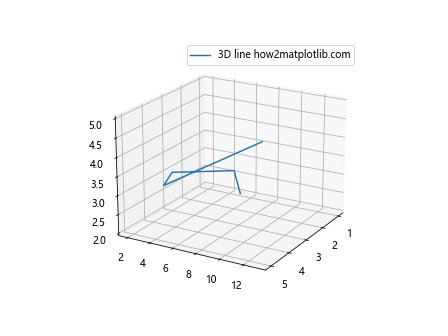
Example 11: Adding Annotations to 3D Points
Annotations can be used to label specific points on your 3D line, providing additional information.
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
x = [1, 2, 3, 4, 5]
y = [5, 6, 2, 3, 13]
z = [2, 3, 3, 3, 5]
ax.plot(x, y, z, label='3D line how2matplotlib.com')
for (i, j, k) in zip(x, y, z):
ax.text(i, j, k, f'({i}, {j}, {k})', color='blue')
ax.legend()
plt.show()
Output:
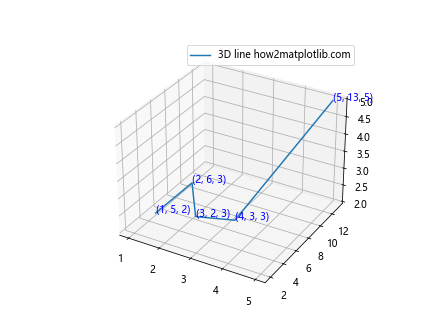
Example 12: Customizing the Background Color
Changing the background color of the plot can enhance the visual appeal of your 3D line.
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d', facecolor='lightgrey')
x = [1, 2, 3, 4, 5]
y = [5, 6, 2, 3, 13]
z = [2, 3, 3, 3, 5]
ax.plot(x, y, z, label='3D line how2matplotlib.com')
ax.legend()
plt.show()
Output:
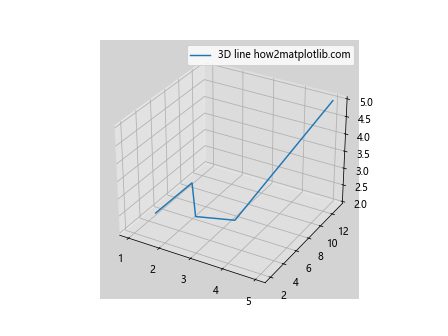
Example 13: Plotting a 3D Line with Transparency
Transparency can be applied to the 3D line to create interesting visual effects.
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
x = [1, 2, 3, 4, 5]
y = [5, 6, 2, 3, 13]
z = [2, 3, 3, 3, 5]
ax.plot(x, y, z, alpha=0.5, label='3D line how2matplotlib.com')
ax.legend()
plt.show()
Output:
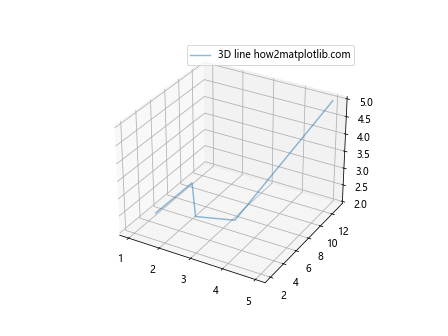
Example 14: Creating a 3D Line with a Legend Outside the Plot
Sometimes it’s useful to place the legend outside the plot to avoid obscuring data.
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
x = [1, 2, 3, 4, 5]
y = [5, 6, 2, 3, 13]
z = [2, 3, 3, 3, 5]
ax.plot(x, y, z, label='3D line how2matplotlib.com')
ax.legend(loc='upper left', bbox_to_anchor=(1, 1))
plt.show()
Output:
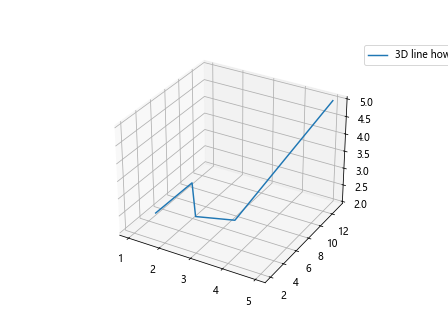
Example 15: Customizing the Plot’s Aspect Ratio
The aspect ratio of the plot can be adjusted to emphasize certain axes.
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d', aspect='auto')
x = [1, 2, 3, 4, 5]
y = [5, 6, 2, 3, 13]
z = [2, 3, 3, 3, 5]
ax.plot(x, y, z, label='3D line how2matplotlib.com')
ax.legend()
plt.show()
Output:
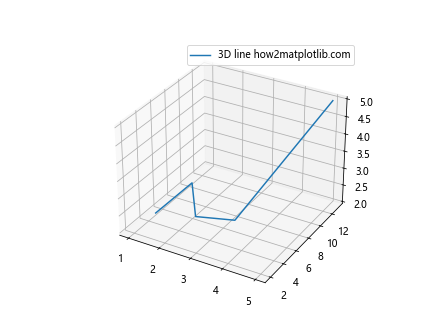
Example 16: Plotting a 3D Line with a Custom Figure Size
The size of the figure can be customized to fit the desired presentation or layout.
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
fig = plt.figure(figsize=(10, 8))
ax = fig.add_subplot(111, projection='3d')
x = [1, 2, 3, 4, 5]
y = [5, 6, 2, 3, 13]
z = [2, 3, 3, 3, 5]
ax.plot(x, y, z, label='3D line how2matplotlib.com')
ax.legend()
plt.show()
Output:
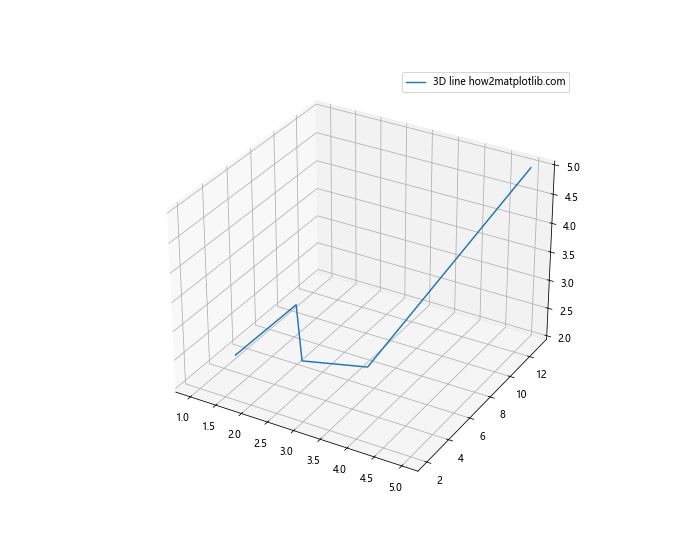