Adjusting Text Background Transparency in Matplotlib
Matplotlib is a powerful plotting library in Python that is used extensively in data visualization. One of the features that can enhance the readability and aesthetics of a plot is the ability to adjust the background transparency of text annotations. This article will explore various ways to adjust text background transparency in Matplotlib, providing comprehensive examples to illustrate each method.
Introduction to Text in Matplotlib
In Matplotlib, text can be added to plots using various functions like text()
, annotate()
, and others. These functions allow customization of the text appearance, including its position, font, color, and importantly, background properties.
Basic Text Properties
Before diving into background transparency, let’s review how to add basic text to a plot in Matplotlib:
Example 1: Adding Basic Text
import matplotlib.pyplot as plt
plt.figure(figsize=(6, 4))
plt.plot([1, 2, 3, 4, 5], [1, 4, 9, 16, 25], label='how2matplotlib.com Example 1')
plt.text(3, 15, 'Basic Text Example', fontsize=12, color='blue')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Basic Text Plot')
plt.legend()
plt.show()
Output:
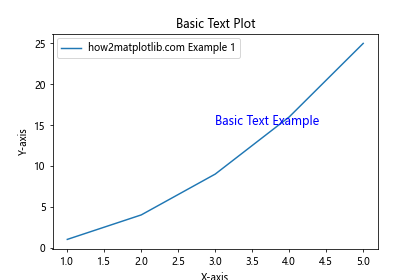
Adjusting Background Color and Transparency
To adjust the background color and transparency of text in Matplotlib, you can use the bbox
property in the text()
function. The bbox
takes a dictionary of rectangle properties.
Example 2: Setting Background Color
import matplotlib.pyplot as plt
plt.figure(figsize=(6, 4))
plt.plot([1, 2, 3, 4, 5], [1, 4, 9, 16, 25], label='how2matplotlib.com Example 2')
plt.text(3, 15, 'Text with Background', fontsize=12, color='black',
bbox=dict(facecolor='yellow', edgecolor='none'))
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Text Background Color')
plt.legend()
plt.show()
Output:
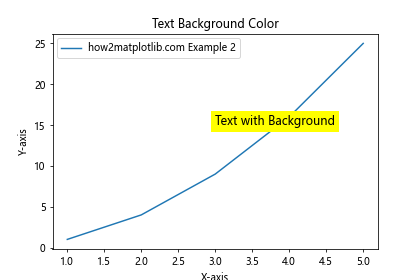
Example 3: Adjusting Background Transparency
import matplotlib.pyplot as plt
plt.figure(figsize=(6, 4))
plt.plot([1, 2, 3, 4, 5], [1, 4, 9, 16, 25], label='how2matplotlib.com Example 3')
plt.text(3, 15, 'Transparent Background Text', fontsize=12, color='black',
bbox=dict(facecolor='red', alpha=0.5))
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Text Background Transparency')
plt.legend()
plt.show()
Output:
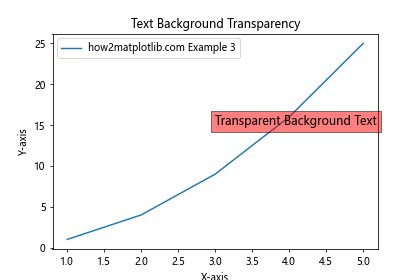
Advanced Text Background Customization
Beyond simple color and transparency, Matplotlib allows for more advanced background customization, such as adding borders, rounding corners, and using gradients.
Example 4: Rounded Corners
import matplotlib.pyplot as plt
plt.figure(figsize=(6, 4))
plt.plot([1, 2, 3, 4, 5], [1, 4, 9, 16, 25], label='how2matplotlib.com Example 4')
plt.text(3, 15, 'Rounded Corners', fontsize=12, color='black',
bbox=dict(facecolor='lightblue', alpha=0.5, boxstyle='round'))
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Rounded Corners Background')
plt.legend()
plt.show()
Output:
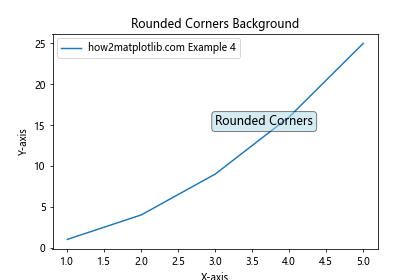
Example 5: Adding a Border
import matplotlib.pyplot as plt
plt.figure(figsize=(6, 4))
plt.plot([1, 2, 3, 4, 5], [1, 4, 9, 16, 25], label='how2matplotlib.com Example 5')
plt.text(3, 15, 'Text with Border', fontsize=12, color='black',
bbox=dict(facecolor='lightgreen', alpha=0.5, edgecolor='black', linewidth=2))
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Text with Border')
plt.legend()
plt.show()
Output:
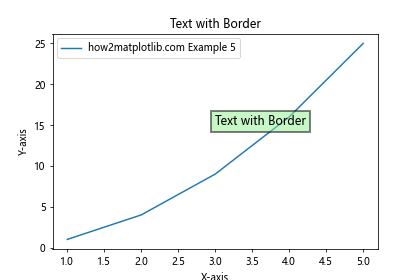
Using Annotations with Background Transparency
Annotations in Matplotlib are similar to text but are typically used to point to a particular part of the plot. They can also have transparent backgrounds.
Example 6: Basic Annotation
import matplotlib.pyplot as plt
plt.figure(figsize=(6, 4))
plt.plot([1, 2, 3, 4, 5], [1, 4, 9, 16, 25], label='how2matplotlib.com Example 6')
plt.annotate('Annotation', xy=(3, 9), xytext=(2, 20),
arrowprops=dict(facecolor='black', shrink=0.05),
bbox=dict(facecolor='orange', alpha=0.5))
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Basic Annotation')
plt.legend()
plt.show()
Output:
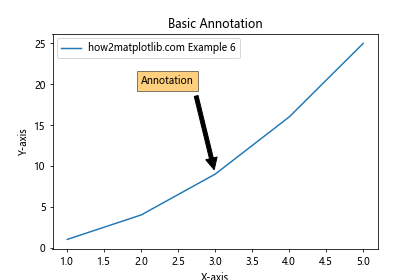
Conclusion
Adjusting the background transparency of text and annotations in Matplotlib can significantly enhance the visual appeal and clarity of plots. By using the bbox
property effectively, you can create visually appealing plots that are easier to interpret and look professional. This guide has provided several examples to help you understand and implement these techniques in your own projects.