Adjust the Width of Box in Boxplot in Python Matplotlib
Creating boxplots is a fundamental technique in data visualization, particularly useful for displaying the distribution of data. In Python, Matplotlib is a widely used library for plotting graphs, and it offers extensive support for creating and customizing boxplots, including adjusting the width of the boxes. This article will explore how to adjust the width of a box in a boxplot using Matplotlib, providing a comprehensive guide with multiple examples.
Introduction to Boxplot
A boxplot, or a box-and-whisper plot, displays the distribution of quantitative data in a way that facilitates comparisons between variables or across levels of a categorical variable. The box shows the quartiles of the dataset while the whiskers extend to show the rest of the distribution, except for points that are determined to be “outliers” using a method that is a function of the inter-quartile range.
Components of a Boxplot:
- Median (Q2/50th Percentile): the middle value of the dataset.
- First quartile (Q1/25th Percentile): the median of the first half of the dataset.
- Third quartile (Q3/75th Percentile): the median of the second half of the dataset.
- Interquartile Range (IQR): the distance between the first and third quartiles.
- Whiskers: the lines that extend from the quartiles to the rest of the data, except for outliers.
- Outliers: points that are distant from other observations.
Adjusting Box Width
The width of the box in a boxplot can be adjusted using the widths
parameter of the boxplot
function in Matplotlib. This parameter can take a scalar or a sequence and is useful for emphasizing comparisons.
Example Code Snippets
Below are several examples of how to create and manipulate the width of boxplots using Matplotlib. Each example is standalone and can be run independently of the others.
Example 1: Basic Boxplot with Custom Width
import matplotlib.pyplot as plt
import numpy as np
# Generate some data
data = np.random.normal(0, 1, 100)
# Create a boxplot
plt.boxplot(data, widths=0.5)
plt.title("Boxplot with Custom Width - how2matplotlib.com")
plt.show()
Output:
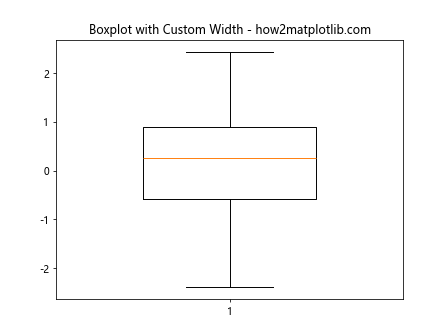
Example 2: Multiple Boxplots with Different Widths
import matplotlib.pyplot as plt
import numpy as np
# Generate data
data1 = np.random.normal(0, 1, 100)
data2 = np.random.normal(0, 2, 100)
# Create multiple boxplots
plt.boxplot([data1, data2], widths=[0.3, 0.5])
plt.title("Multiple Boxplots with Different Widths - how2matplotlib.com")
plt.show()
Output:
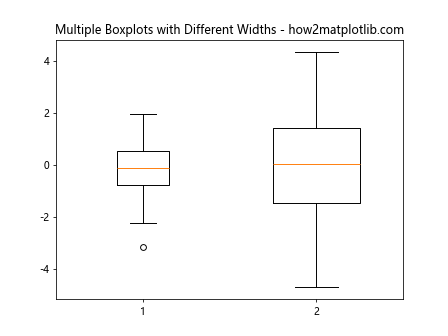
Example 3: Boxplot with Extreme Width
import matplotlib.pyplot as plt
import numpy as np
# Generate data
data = np.random.normal(0, 1, 100)
# Create a boxplot with extreme width
plt.boxplot(data, widths=1.0)
plt.title("Boxplot with Extreme Width - how2matplotlib.com")
plt.show()
Output:
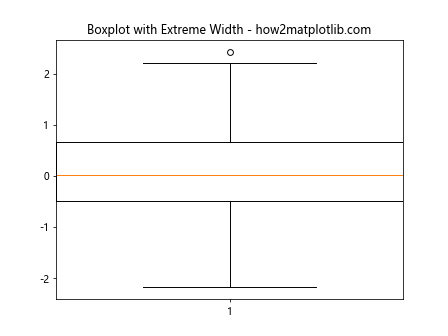
Example 4: Boxplot with Very Narrow Width
import matplotlib.pyplot as plt
import numpy as np
# Generate data
data = np.random.normal(0, 1, 100)
# Create a boxplot with very narrow width
plt.boxplot(data, widths=0.1)
plt.title("Boxplot with Very Narrow Width - how2matplotlib.com")
plt.show()
Output:
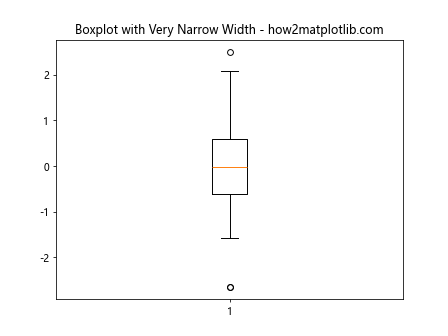
Example 5: Boxplots with Sequentially Increasing Widths
import matplotlib.pyplot as plt
import numpy as np
# Generate data
data1 = np.random.normal(0, 1, 100)
data2 = np.random.normal(0, 2, 100)
data3 = np.random.normal(0, 3, 100)
# Create boxplots with sequentially increasing widths
plt.boxplot([data1, data2, data3], widths=[0.2, 0.4, 0.6])
plt.title("Boxplots with Sequentially Increasing Widths - how2matplotlib.com")
plt.show()
Output:
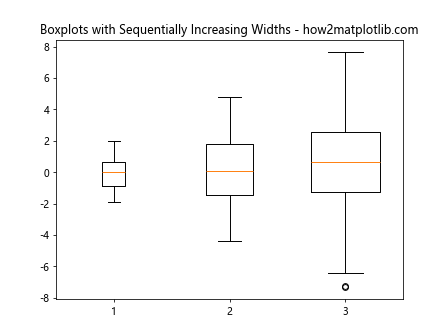
Example 6: Boxplot with Custom Outlier Symbols and Width
import matplotlib.pyplot as plt
import numpy as np
# Generate data
data = np.random.normal(0, 1, 100)
# Create a boxplot with custom outlier symbols and width
plt.boxplot(data, widths=0.4, flierprops=dict(marker='o', color='red', markersize=12))
plt.title("Boxplot with Custom Outlier Symbols and Width - how2matplotlib.com")
plt.show()
Output:
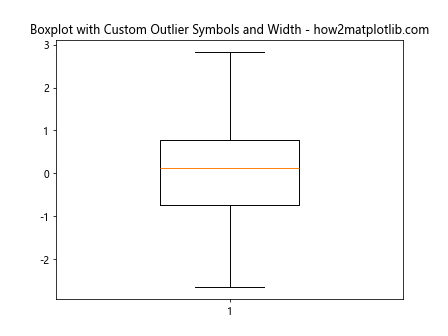
Example 7: Horizontal Boxplot with Custom Width
import matplotlib.pyplot as plt
import numpy as np
# Generate data
data = np.random.normal(0, 1, 100)
# Create a horizontal boxplot with custom width
plt.boxplot(data, widths=0.4, vert=False)
plt.title("Horizontal Boxplot with Custom Width - how2matplotlib.com")
plt.show()
Output:
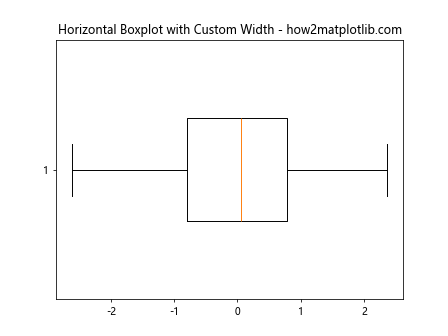
Example 8: Boxplot with Custom Colors and Width
import matplotlib.pyplot as plt
import numpy as np
# Generate data
data = np.random.normal(0, 1, 100)
# Create a boxplot with custom colors and width
plt.boxplot(data, widths=0.5, patch_artist=True, boxprops=dict(facecolor='lightblue'))
plt.title("Boxplot with Custom Colors and Width - how2matplotlib.com")
plt.show()
Output:
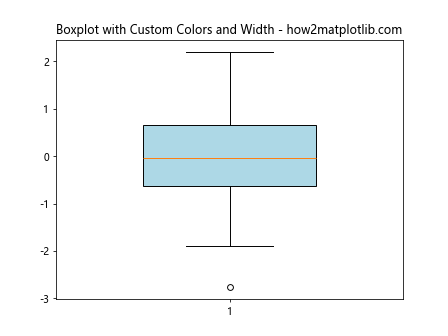
Example 9: Boxplot with Different Widths for Each Box
import matplotlib.pyplot as plt
import numpy as np
# Generate data
data1 = np.random.normal(0, 1, 100)
data2 = np.random.normal(0, 2, 100)
data3 = np.random.normal(0, 3, 100)
# Create boxplots with different widths for each box
plt.boxplot([data1, data2, data3], widths=[0.1, 0.5, 0.9])
plt.title("Boxplot with Different Widths for Each Box - how2matplotlib.com")
plt.show()
Output:
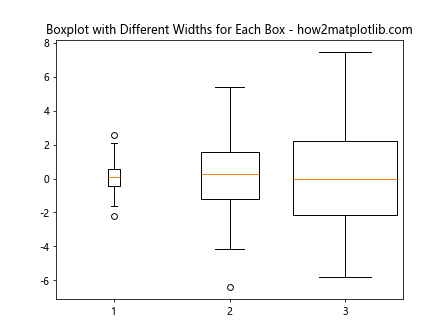
Example 10: Boxplot with Width and Custom Whisker Length
import matplotlib.pyplot as plt
import numpy as np
# Generate data
data = np.random.normal(0, 1, 100)
# Create a boxplot with width and custom whisker length
plt.boxplot(data, widths=0.5, whiskerprops=dict(linestyle='--', linewidth=2))
plt.title("Boxplot with Width and Custom Whisker Length - how2matplotlib.com")
plt.show()
Output:
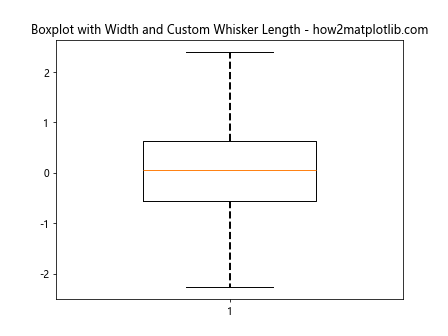
Conclusion
Adjusting the width of the boxes in a boxplot using Matplotlib is a straightforward process that can significantly enhance the readability and aesthetics of your plots. By using the widths
parameter, you can tailor the appearance of your boxplots to better convey the message of your data visualization. The examples provided in this article demonstrate various ways to customize the width along with other aspects of boxplots, offering a robust foundation for creating compelling visualizations with Python’s Matplotlib library.