Adding Caption Below X-axis for a Scatter Plot Using Matplotlib
Creating effective visualizations is crucial for data analysis and communication. Among the various tools available for data visualization in Python, Matplotlib is one of the most popular and widely used libraries. It provides a powerful way to generate a wide range of plots and charts with high degree of customization. In this article, we will focus on how to add captions below the X-axis of a scatter plot using Matplotlib. This can be particularly useful for providing additional information about the data or the plot itself.
Introduction to Matplotlib
Matplotlib is a plotting library for the Python programming language and its numerical mathematics extension NumPy. It provides an object-oriented API for embedding plots into applications using general-purpose GUI toolkits like Tkinter, wxPython, Qt, or GTK. Before diving into the specifics of adding captions, let’s first discuss how to set up Matplotlib and create a basic scatter plot.
Setting Up Matplotlib
To get started with Matplotlib, you need to install the package using pip if it’s not already installed:
pip install matplotlib
Once installed, you can import it in your Python script:
import matplotlib.pyplot as as plt
Creating a Basic Scatter Plot
Here’s a simple example of creating a scatter plot:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
plt.scatter(x, y)
plt.title("Simple Scatter Plot - how2matplotlib.com")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
plt.show()
Output:
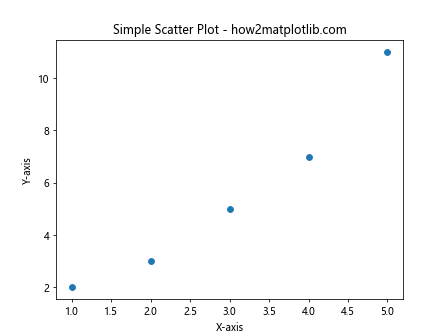
Adding Captions Below the X-axis
Adding captions below the X-axis in a scatter plot can be achieved by using the text
function in Matplotlib, which allows you to place text at an arbitrary location on the Axes. This function also lets you customize the text properties to fit the style of your plot.
Example 1: Basic Caption
Here’s how you can add a basic caption below the X-axis:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
plt.scatter(x, y)
plt.title("Scatter Plot with Caption - how2matplotlib.com")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
plt.text(2.5, 1, "This is a caption below the X-axis - how2matplotlib.com", ha='center')
plt.show()
Output:
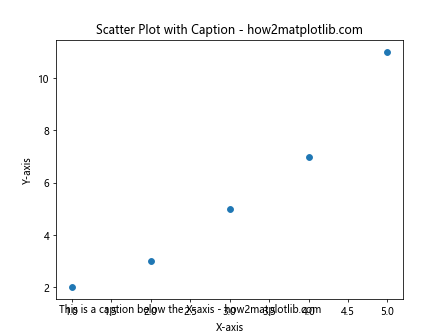
Example 2: Caption with Styling
You can also style the caption by adjusting the font properties:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
plt.scatter(x, y)
plt.title("Styled Caption - how2matplotlib.com")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
plt.text(2.5, 1, "Styled caption below X-axis - how2matplotlib.com", ha='center', fontweight='bold', color='red')
plt.show()
Output:
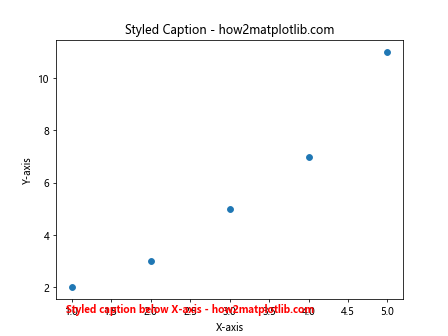
Example 3: Caption with Multiple Lines
If you need to add a longer caption, you can use multiple lines:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
plt.scatter(x, y)
plt.title("Multi-line Caption - how2matplotlib.com")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
plt.text(2.5, 0.5, "This is a multi-line\ncaption below the X-axis - how2matplotlib.com", ha='center')
plt.show()
Output:
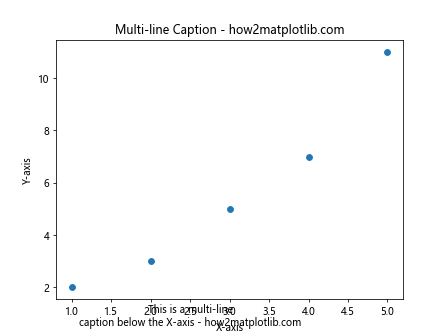
Example 4: Adjusting Caption Position
Adjusting the position of the caption relative to the X-axis can be done by modifying the y-coordinate in the text
function:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
plt.scatter(x, y)
plt.title("Adjusted Caption Position - how2matplotlib.com")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
plt.text(2.5, -1, "Caption with adjusted position - how2matplotlib.com", ha='center')
plt.show()
Output:
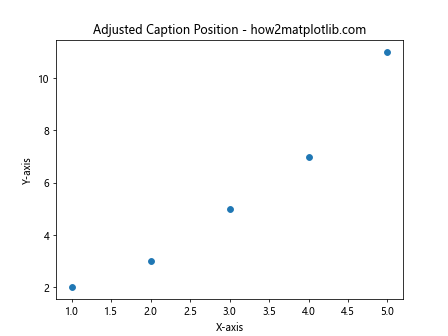
Example 5: Caption with Mathematical Expressions
Matplotlib supports LaTeX syntax for mathematical expressions in any text element. Here’s how to include a mathematical expression in your caption:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
plt.scatter(x, y)
plt.title("Caption with Math - how2matplotlib.com")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
plt.text(2.5, 1, r'$\alpha > \beta$ caption below X-axis - how2matplotlib.com', ha='center')
plt.show()
Output:
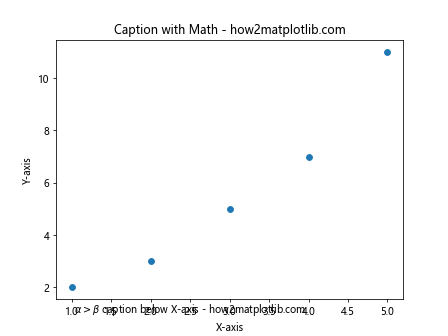
Conclusion
In this article, we explored various ways to add captions below the X-axis of a scatter plot using Matplotlib. We covered basic captions, styled captions, multi-line captions, adjusted positions, and even mathematical expressions. These techniques enhance the readability and informativeness of your plots, making them more useful for presentations or academic papers.