Add Alpha to an Existing Matplotlib Colormap
Adding alpha (transparency) to an existing colormap in Matplotlib can be a powerful way to enhance the visual appeal and clarity of plots, especially when dealing with overlapping data or creating overlays. Matplotlib, a comprehensive library for creating static, animated, and interactive visualizations in Python, offers extensive support for customizing colormaps. In this article, we will explore various methods to add alpha values to existing colormaps and demonstrate these techniques with detailed examples.
Understanding Colormaps and Alpha
A colormap in Matplotlib is essentially a series of colors, where each color corresponds to a data value. The alpha value represents the transparency of the color, where 0 is completely transparent (invisible) and 1 is completely opaque.
Why Add Alpha?
Adding alpha to colormaps can be useful in several scenarios:
- Overlapping Plots: Improve clarity when multiple layers overlap in a plot.
- Highlighting: Emphasize specific regions of a plot while de-emphasizing others.
- Aesthetics: Enhance the visual appeal of plots with subtle transparency effects.
Methods to Add Alpha to Colormaps
There are several approaches to add alpha values to colormaps in Matplotlib. We will explore each method and provide example code for implementation.
Method 1: Using the cmap
and alpha
Parameters
The simplest way to add alpha to a plot is by using the alpha
parameter available in most plotting functions. However, this method applies a uniform alpha across all colors.
Example Code 1
import matplotlib.pyplot as plt
import numpy as np
data = np.random.rand(10,10)
plt.imshow(data, cmap='viridis', alpha=0.5)
plt.title("Uniform Alpha - how2matplotlib.com")
plt.colorbar()
plt.show()
Output:
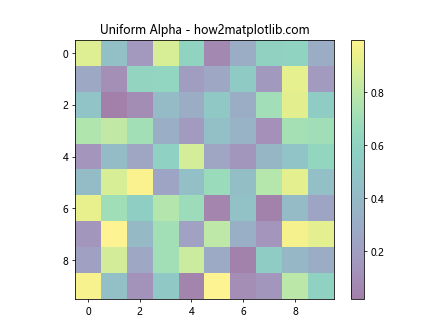
Method 2: Modifying the Colormap Directly
For more control, you can modify the colormap directly to include alpha values. This method involves creating a new colormap from the existing one by appending alpha values to the RGBA tuples.
Example Code 2
import matplotlib.pyplot as plt
import numpy as np
import matplotlib.colors as mcolors
data = np.random.rand(10,10)
cmap = plt.cm.Blues
new_cmap = mcolors.ListedColormap([(r, g, b, 0.5) for r, g, b, _ in cmap(np.arange(cmap.N))])
plt.imshow(data, cmap=new_cmap)
plt.title("Modified Colormap with Alpha - how2matplotlib.com")
plt.colorbar()
plt.show()
Output:
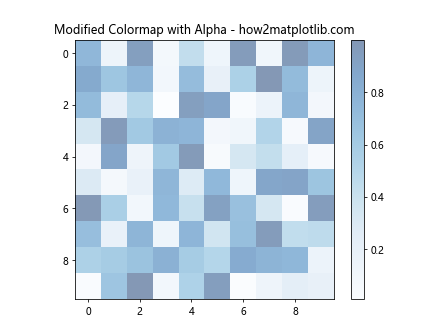
Method 3: Using LinearSegmentedColormap
For a gradient-based approach, you can use LinearSegmentedColormap
to interpolate between colors while incorporating alpha values.
Example Code 3
import matplotlib.pyplot as plt
import numpy as np
import matplotlib.colors as mcolors
data = np.random.rand(10,10)
colors = [(1, 0, 0, 0), (1, 0, 0, 1)] # Red color with gradient alpha
cmap = mcolors.LinearSegmentedColormap.from_list("RedAlpha", colors)
plt.imshow(data, cmap=cmap)
plt.title("Gradient Alpha with LinearSegmentedColormap - how2matplotlib.com")
plt.colorbar()
plt.show()
Output:
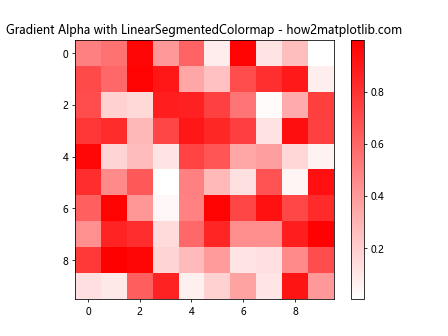
Method 4: Creating a Custom Colormap with Transparency
For full control, you can define a custom colormap including alpha values using from_list
method of ListedColormap
.
Example Code 4
import matplotlib.pyplot as plt
import numpy as np
import matplotlib.colors as mcolors
data = np.random.rand(10,10)
colors = [(0, 1, 0, i/10) for i in range(10)] # Green with increasing alpha
cmap = mcolors.ListedColormap(colors, name='GreenAlpha')
plt.imshow(data, cmap=cmap)
plt.title("Custom Colormap with Increasing Alpha - how2matplotlib.com")
plt.colorbar()
plt.show()
Output:
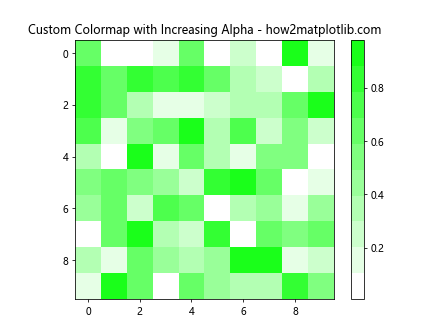
Method 5: Using Normalize with Alpha
Sometimes, you might want to adjust the alpha level based on the data values. This can be achieved by using a normalization function along with a modified colormap.
Example Code 5
import matplotlib.pyplot as plt
import numpy as np
import matplotlib.colors as mcolors
data = np.random.rand(10,10)
norm = mcolors.Normalize(vmin=0, vmax=1)
colors = [(0, 0, 1, norm(value)) for value in np.linspace(0, 1, 256)] # Blue with alpha based on value
cmap = mcolors.ListedColormap(colors)
plt.imshow(data, cmap=cmap)
plt.title("Alpha Based on Data Value - how2matplotlib.com")
plt.colorbar()
plt.show()
Output:
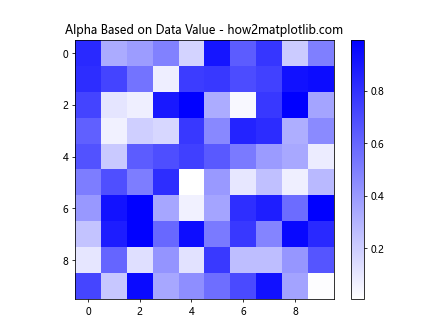
Conclusion
Adding alpha to colormaps in Matplotlib can significantly enhance the visualization by providing depth, clarity, and aesthetic appeal to the plots. By using the methods described above, you can customize the transparency of your plots to suit specific requirements and improve the overall presentation of data. Whether you are dealing with overlapping data points, creating thematic maps, or simply looking to create visually appealing charts, these techniques provide the tools necessary to achieve your objectives.
This guide has provided a comprehensive overview of how to add alpha to existing colormaps in Matplotlib, complete with practical examples that can be directly applied or adapted to your own projects. Remember, the key to effective visualization is not only in representing data accurately but also in making it accessible and engaging for the audience. Transparency is just one of the many tools at your disposal to achieve this goal.