Comprehensive Guide to Using Matplotlib.axis.Tick.get_gid() in Python
Matplotlib.axis.Tick.get_gid() in Python is an essential method for working with tick objects in Matplotlib, a popular data visualization library. This article will explore the various aspects of using Matplotlib.axis.Tick.get_gid() in Python, providing detailed explanations and practical examples to help you master this powerful feature.
Understanding Matplotlib.axis.Tick.get_gid() in Python
Matplotlib.axis.Tick.get_gid() in Python is a method that belongs to the Tick class in Matplotlib’s axis module. It is used to retrieve the group id (gid) of a tick object. The gid is a string that can be used to identify and group related elements in a Matplotlib plot.
Let’s start with a simple example to demonstrate how to use Matplotlib.axis.Tick.get_gid() in Python:
import matplotlib.pyplot as plt
# Create a figure and axis
fig, ax = plt.subplots()
# Plot some data
ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='Data from how2matplotlib.com')
# Get the x-axis ticks
x_ticks = ax.get_xticks()
# Get the gid of the first x-axis tick
first_tick = ax.xaxis.get_major_ticks()[0]
gid = first_tick.get_gid()
print(f"GID of the first x-axis tick: {gid}")
plt.show()
Output:
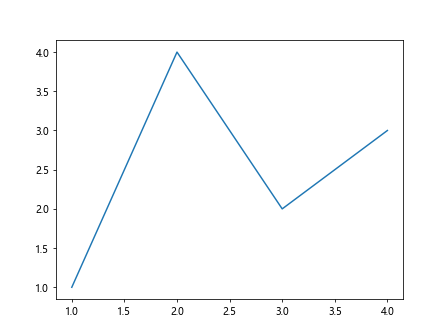
In this example, we create a simple line plot and then use Matplotlib.axis.Tick.get_gid() in Python to retrieve the gid of the first x-axis tick. The gid is typically None by default unless it has been explicitly set.
Setting and Getting GIDs with Matplotlib.axis.Tick.get_gid() in Python
To make better use of Matplotlib.axis.Tick.get_gid() in Python, we often need to set gids for tick objects first. Let’s explore how to do this:
import matplotlib.pyplot as plt
# Create a figure and axis
fig, ax = plt.subplots()
# Plot some data
ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='Data from how2matplotlib.com')
# Set gids for x-axis ticks
for i, tick in enumerate(ax.xaxis.get_major_ticks()):
tick.set_gid(f"xtick_{i}")
# Get and print gids of all x-axis ticks
for i, tick in enumerate(ax.xaxis.get_major_ticks()):
gid = tick.get_gid()
print(f"GID of x-axis tick {i}: {gid}")
plt.show()
Output:
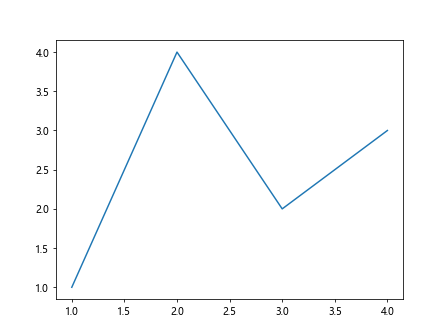
In this example, we set unique gids for each x-axis tick using a loop and the set_gid() method. Then, we use Matplotlib.axis.Tick.get_gid() in Python to retrieve and print the gids of all x-axis ticks.
Practical Applications of Matplotlib.axis.Tick.get_gid() in Python
Matplotlib.axis.Tick.get_gid() in Python can be particularly useful when you need to identify and manipulate specific ticks in your plots. Here’s an example that demonstrates how to use gids to style specific ticks:
import matplotlib.pyplot as plt
# Create a figure and axis
fig, ax = plt.subplots()
# Plot some data
ax.plot([1, 2, 3, 4, 5], [2, 4, 1, 3, 5], label='Data from how2matplotlib.com')
# Set gids for x-axis ticks
for i, tick in enumerate(ax.xaxis.get_major_ticks()):
tick.set_gid(f"xtick_{i}")
# Style specific ticks based on their gids
for tick in ax.xaxis.get_major_ticks():
if tick.get_gid() in ["xtick_1", "xtick_3"]:
tick.label1.set_color('red')
tick.label1.set_fontweight('bold')
plt.show()
Output:
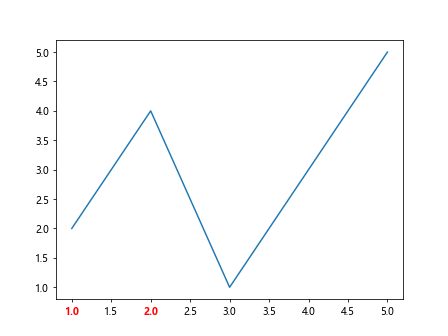
In this example, we use Matplotlib.axis.Tick.get_gid() in Python to identify specific ticks (the second and fourth ticks) and style them differently by changing their color and font weight.
Advanced Usage of Matplotlib.axis.Tick.get_gid() in Python
Matplotlib.axis.Tick.get_gid() in Python can be combined with other Matplotlib features to create more complex and interactive visualizations. Let’s explore some advanced applications:
Using Matplotlib.axis.Tick.get_gid() with Event Handling
import matplotlib.pyplot as plt
def on_click(event):
if event.inaxes:
for tick in event.inaxes.xaxis.get_major_ticks():
if tick.get_gid() == "clickable":
print(f"Clicked near tick with value: {tick.get_loc()}")
# Create a figure and axis
fig, ax = plt.subplots()
# Plot some data
ax.plot([1, 2, 3, 4, 5], [2, 4, 1, 3, 5], label='Data from how2matplotlib.com')
# Set gids for x-axis ticks
for i, tick in enumerate(ax.xaxis.get_major_ticks()):
if i % 2 == 0:
tick.set_gid("clickable")
# Connect the click event to the on_click function
fig.canvas.mpl_connect('button_press_event', on_click)
plt.show()
Output:
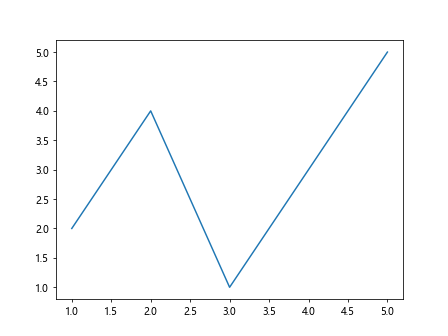
In this example, we use Matplotlib.axis.Tick.get_gid() in Python to make every other tick “clickable”. When a user clicks near a tick with the “clickable” gid, the program prints the tick’s value.
Combining Matplotlib.axis.Tick.get_gid() with Custom Tick Formatters
import matplotlib.pyplot as plt
import matplotlib.ticker as ticker
class CustomFormatter(ticker.Formatter):
def __call__(self, x, pos=None):
tick = self.axis.get_major_ticks()[pos]
if tick.get_gid() == "highlight":
return f"**{x:.1f}**"
return f"{x:.1f}"
# Create a figure and axis
fig, ax = plt.subplots()
# Plot some data
ax.plot([1, 2, 3, 4, 5], [2, 4, 1, 3, 5], label='Data from how2matplotlib.com')
# Set gids for x-axis ticks
for i, tick in enumerate(ax.xaxis.get_major_ticks()):
if i % 2 == 0:
tick.set_gid("highlight")
# Set the custom formatter
ax.xaxis.set_major_formatter(CustomFormatter())
plt.show()
Output:
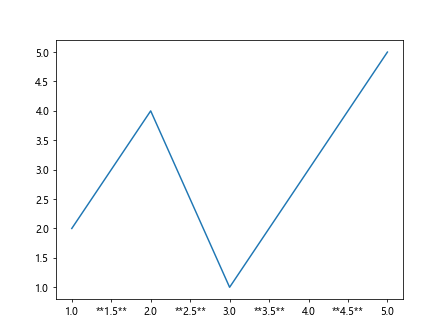
In this example, we create a custom tick formatter that uses Matplotlib.axis.Tick.get_gid() in Python to format ticks differently based on their gid. Ticks with the “highlight” gid are surrounded by asterisks.
Best Practices for Using Matplotlib.axis.Tick.get_gid() in Python
When working with Matplotlib.axis.Tick.get_gid() in Python, it’s important to follow some best practices to ensure your code is efficient and maintainable:
- Set meaningful gids: Choose gid names that clearly describe the purpose or characteristic of the tick.
Use consistent naming conventions: If you’re setting gids for multiple elements, use a consistent naming scheme.
Check for None: Remember that get_gid() may return None if no gid has been set.
Combine with other Matplotlib features: Matplotlib.axis.Tick.get_gid() in Python is most powerful when used in conjunction with other Matplotlib methods and properties.
Here’s an example that demonstrates these best practices: