Annotate Subplots in a Figure with A, B, C using Matplotlib
Annotating subplots in a figure with labels such as A, B, C is a common practice in scientific publications to provide clear references to specific plots within a figure. This technique helps in discussing and referencing these plots in the text of articles, papers, or reports. Matplotlib, a powerful plotting library in Python, offers various ways to annotate subplots effectively. In this article, we will explore different methods to annotate subplots in a figure using Matplotlib, providing comprehensive examples to illustrate each approach.
Introduction to Matplotlib
Matplotlib is a widely used Python library for creating static, interactive, and animated visualizations in Python. It provides an object-oriented API that allows users to embed plots into applications using general-purpose GUI toolkits like Tkinter, wxPython, Qt, or GTK. Before diving into the specific methods of annotating subplots, it’s essential to understand some basics of Matplotlib.
Basic Plotting
Here’s a simple example of how to create a basic plot in Matplotlib:
import matplotlib.pyplot as plt
# Data
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
# Create a figure and an axes.
fig, ax = plt.subplots()
# Plotting the data
ax.plot(x, y, label='Data Line')
# Adding a title and labels
ax.set_title('Basic Plot - how2matplotlib.com')
ax.set_xlabel('X Axis')
ax.set_ylabel('Y Axis')
# Show legend
ax.legend()
# Display the plot
plt.show()
Output:
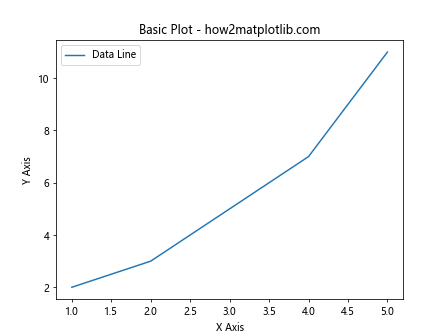
Annotating Subplots
When dealing with multiple subplots, it’s often useful to label each subplot for better clarity. Below are various methods to annotate subplots in a figure.
Method 1: Using text
Function
The text
function in Matplotlib allows you to add text at any position on the plot. Here’s how you can use it to annotate subplots.
import matplotlib.pyplot as plt
# Create a figure with 2 subplots
fig, (ax1, ax2) = plt.subplots(1, 2)
# First subplot
ax1.plot([1, 2, 3], [1, 4, 9], 'r-')
ax1.set_title('Plot 1 - how2matplotlib.com')
ax1.text(0.5, 0.5, 'A', fontsize=20, ha='center', va='center', transform=ax1.transAxes)
# Second subplot
ax2.plot([1, 2, 3], [9, 4, 1], 'b-')
ax2.set_title('Plot 2 - how2matplotlib.com')
ax2.text(0.5, 0.5, 'B', fontsize=20, ha='center', va='center', transform=ax2.transAxes)
# Show the figure
plt.show()
Output:
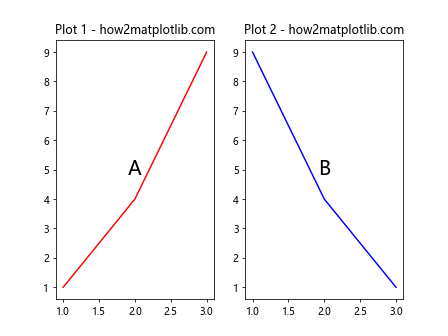
Method 2: Using annotate
Function
The annotate
function provides more flexibility compared to text
for adding annotations, as it can also draw arrows.
import matplotlib.pyplot as plt
# Create a figure with 2 subplots
fig, (ax1, ax2) = plt.subplots(1, 2)
# First subplot
ax1.plot([1, 2, 3], [1, 4, 9], 'r-')
ax1.set_title('Plot 1 - how2matplotlib.com')
ax1.annotate('A', xy=(0.5, 0.5), xycoords='axes fraction', fontsize=20, ha='center')
# Second subplot
ax2.plot([1, 2, 3], [9, 4, 1], 'b-')
ax2.set_title('Plot 2 - how2matplotlib.com')
ax2.annotate('B', xy=(0.5, 0.5), xycoords='axes fraction', fontsize=20, ha='center')
# Show the figure
plt.show()
Output:
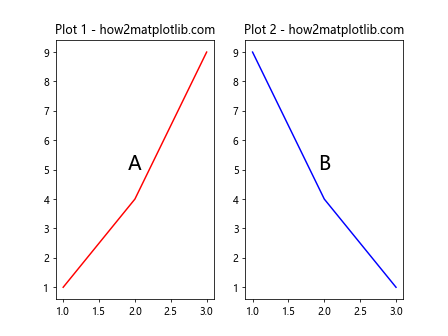
Method 3: Using figtext
Function
figtext
places text at a position relative to the whole figure, which can be useful when your annotations need to be placed outside the subplots.
import matplotlib.pyplot as plt
# Create a figure with 2 subplots
fig, (ax1, ax2) = plt.subplots(1, 2)
# First subplot
ax1.plot([1, 2, 3], [1, 4, 9], 'r-')
ax1.set_title('Plot 1 - how2matplotlib.com')
# Second subplot
ax2.plot([1, 2, 3], [9, 4, 1], 'b-')
ax2.set_title('Plot 2 - how2matplotlib.com')
# Adding annotations with figtext
plt.figtext(0.25, 0.5, 'A', fontsize=20, ha='center')
plt.figtext(0.75, 0.5, 'B', fontsize=20, ha='center')
# Show the figure
plt.show()
Output:
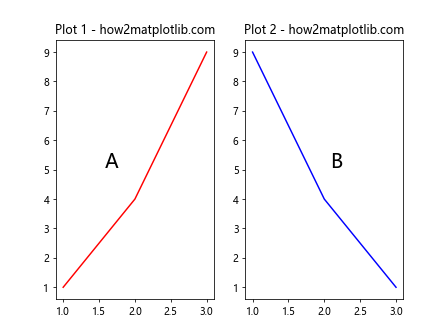
Method 4: Using Custom Function for Automatic Labeling
For more complex layouts or a large number of subplots, it might be practical to create a custom function to automate the labeling process.
import matplotlib.pyplot as plt
import numpy as np
def label_subplots(axs, labels=['A', 'B', 'C', 'D'], xpos=-0.1, ypos=1.1):
for ax, label in zip(axs, labels):
ax.text(xpos, ypos, label, transform=ax.transAxes, fontsize=16, fontweight='bold', va='top', ha='right')
# Create a 2x2 grid of subplots
fig, axs = plt.subplots(2, 2)
axs = axs.flatten()
# Plotting some data
for ax in axs:
ax.plot(np.random.rand(10), np.random.rand(10), 'o-')
# Labeling subplots
label_subplots(axs, xpos=0.1, ypos=0.9)
# Adjust layout
plt.tight_layout()
# Show the figure
plt.show()
Output:
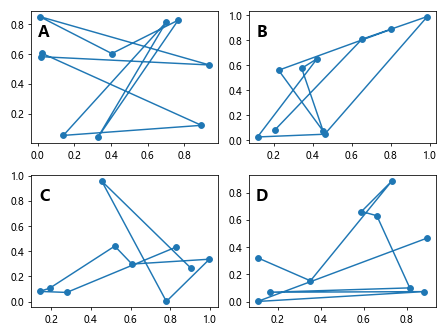
Conclusion
Labeling subplots in a figure is essential for clear communication in scientific publications and reports. Matplotlib provides various tools and functions to facilitate this, making it a versatile choice for creating annotated plots. By using the methods described above, you can effectively annotate your subplots in any figure layout.
This guide has provided a comprehensive overview of annotating subplots in Matplotlib, complete with detailed examples. Whether you are preparing a publication or simply organizing your data visualization for better clarity, these techniques will surely enhance your plotting skills.