Subplot Title in Matplotlib
In Matplotlib, subplots are useful for displaying multiple plots within the same figure. Each subplot can have its own individual title to provide additional information about the data being displayed. In this article, we will explore how to set titles for subplots in Matplotlib.
Setting Subplot Titles
We can set titles for subplots using the set_title()
method of the subplot object. This method allows us to specify the title text, fontsize, color, and other properties of the subplot title.
import matplotlib.pyplot as plt
# Create a figure and axis to hold subplots
fig, axs = plt.subplots(2, 2)
# Set title for subplot at position (0,0)
axs[0, 0].set_title('Subplot 1', fontsize=12, color='blue')
# Set title for subplot at position (0,1)
axs[0, 1].set_title('Subplot 2', fontsize=10, color='green')
plt.show()
Output:
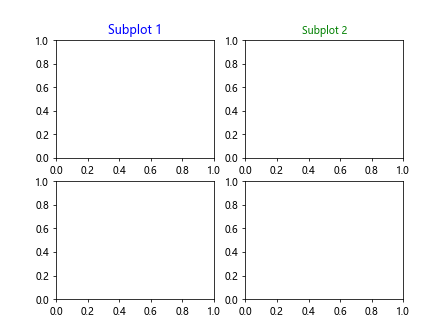
In the example above, we create a 2×2 grid of subplots and set titles for each of the subplots. The titles are customized with different font sizes and colors.
Adding Subplot Title with Bold Text
We can enhance the appearance of the subplot title by making the text bold. This can be achieved by setting the fontweight
property of the title.
import matplotlib.pyplot as plt
# Create a figure and axis to hold subplots
fig, axs = plt.subplots()
# Set title for subplot with bold text
axs.set_title('Bold Subplot Title', fontsize=14, fontweight='bold')
plt.show()
Output:
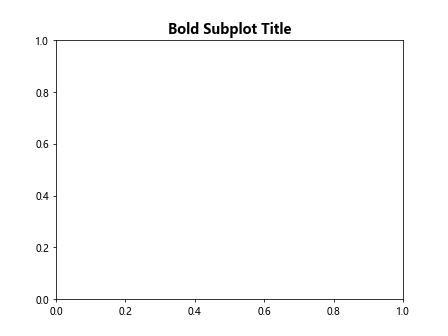
In the code snippet above, we set the title of the subplot with bold text by specifying the fontweight
property as 'bold'
.
Centering Subplot Title
By default, the subplot title is placed at the left of the subplot. We can center the title by adjusting the alignment using the horizontalalignment
property.
import matplotlib.pyplot as plt
# Create a figure and axis to hold subplots
fig, axs = plt.subplots()
# Center the title of the subplot
axs.set_title('Centered Subplot Title', fontsize=14, horizontalalignment='center')
plt.show()
Output:
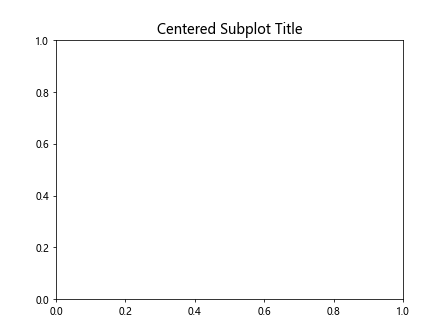
In the example above, we use the horizontalalignment
property with the value 'center'
to center the title of the subplot.
Adding Subplot Title with Background Color
We can highlight the subplot title by adding a background color to it. This can be done by setting the bbox
property of the title.
import matplotlib.pyplot as plt
# Create a figure and axis to hold subplots
fig, axs = plt.subplots()
# Add background color to the title of the subplot
axs.set_title('Subplot Title with Background Color', fontsize=14, bbox={'facecolor': 'yellow', 'alpha': 0.5})
plt.show()
Output:
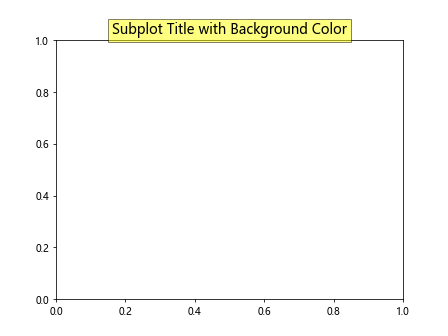
In the code above, we specify the facecolor
property of the bbox
parameter to set the background color of the subplot title.
Changing Font Family of Subplot Title
We can change the font family of the subplot title to customize its appearance. This can be accomplished by setting the fontname
property of the title.
import matplotlib.pyplot as plt
# Create a figure and axis to hold subplots
fig, axs = plt.subplots()
# Change the font family of the subplot title
axs.set_title('Subplot Title with Custom Font', fontsize=14, fontname='Comic Sans MS')
plt.show()
Output:
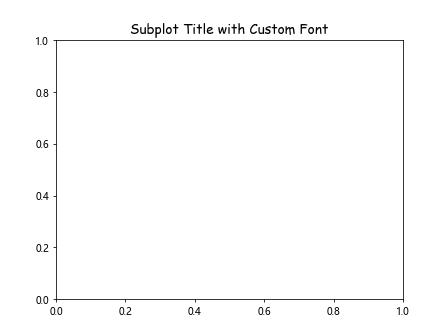
In the code snippet above, we change the font family of the subplot title to ‘Comic Sans MS’ by specifying the fontname
property.
Rotating Subplot Title
We can rotate the subplot title to a specific angle to change its orientation using the rotation
property.
import matplotlib.pyplot as plt
# Create a figure and axis to hold subplots
fig, axs = plt.subplots()
# Rotate the title of the subplot
axs.set_title('Rotated Subplot Title', fontsize=16, rotation=45)
plt.show()
Output:
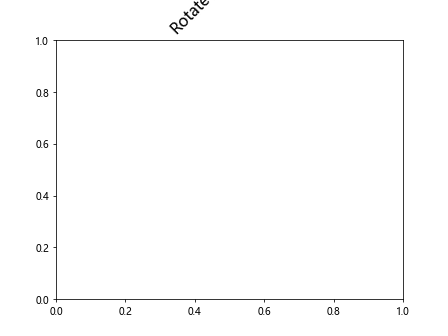
In the example above, we set the rotation angle of the subplot title to 45 degrees using the rotation
property.
Subplot Title with Wrap Text
Sometimes, the subplot title may be too long to fit within the available space. We can wrap the text of the title by setting the wrap
property to True.
import matplotlib.pyplot as plt
# Create a figure and axis to hold subplots
fig, axs = plt.subplots()
# Wrap the text of the subplot title
axs.set_title('This is a long subplot title that needs to be wrapped', fontsize=14, wrap=True)
plt.show()
Output:
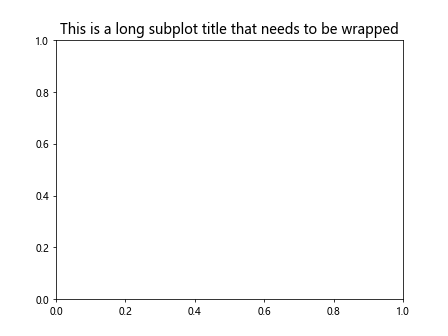
In the code snippet above, we enable text wrapping for the subplot title by setting the wrap
property to True.
Subplot Title with Multiple Lines
We can create a subplot title with multiple lines by using the newline character \n
to separate the lines of text.
import matplotlib.pyplot as plt
# Create a figure and axis to hold subplots
fig, axs = plt.subplots()
# Create a title with multiple lines
axs.set_title('First Line\nSecond Line', fontsize=14)
plt.show()
Output:
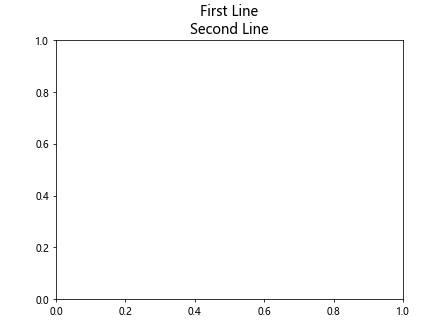
In the example above, we use the newline character \n
to create a subplot title with two lines of text.
Subplot Title with Math Symbols
Matplotlib allows us to include math symbols in the subplot title by using LaTeX notation. We need to enclose the math symbol within $
symbols.
import matplotlib.pyplot as plt
# Create a figure and axis to hold subplots
fig, axs = plt.subplots()
# Add math symbols to the title
axs.set_title('$\int_0^1 x^2 dx$', fontsize=14)
plt.show()
In the code snippet above, we use LaTeX notation to add the integral symbol to the subplot title.
Subplot Title with Unicode Characters
We can include Unicode characters in the subplot title to add special symbols or emojis.
import matplotlib.pyplot as plt
# Create a figure and axis to hold subplots
fig, axs = plt.subplots()
# Add Unicode character to the title
axs.set_title('Subplot Title with Emoji 🎉', fontsize=14)
plt.show()
In the example above, we include the emoji 🎉 as a Unicode character in the subplot title.
Conclusion
In this article, we have explored various ways to customize subplot titles in Matplotlib. By setting different properties such as font size, color, font family, rotation angle, and background color, we can enhance the appearance of the subplot titles. We have also demonstrated how to include math symbols, special characters, and emojis in the subplot titles to make them more informative and visually appealing. Experiment with these techniques to create visually engaging plots with descriptive subplot titles in Matplotlib.